haystack-tutorials
5015 строк · 150.6 Кб
1{
2"cells": [
3{
4"attachments": {},
5"cell_type": "markdown",
6"metadata": {
7"collapsed": false,
8"id": "Oo2_F2jwsA8J",
9"pycharm": {
10"name": "#%% md\n"
11}
12},
13"source": [
14"# Tutorial: How to Use Pipelines\n",
15"\n",
16"In this tutorial, you will learn how the `Pipeline` connects the different components in Haystack. Whether you are using a Reader, Summarizer\n",
17"or Retriever (or 2), the `Pipeline` class will help you build a Directed Acyclic Graph (DAG) that\n",
18"determines how to route the output of one component into the input of another.\n"
19]
20},
21{
22"attachments": {},
23"cell_type": "markdown",
24"metadata": {
25"collapsed": false,
26"id": "DwGR2m4MsA8K",
27"pycharm": {
28"name": "#%% md\n"
29}
30},
31"source": [
32"\n",
33"## Preparing the Colab Environment\n",
34"\n",
35"- [Enable GPU Runtime](https://docs.haystack.deepset.ai/docs/enabling-gpu-acceleration#enabling-the-gpu-in-colab)\n"
36]
37},
38{
39"attachments": {},
40"cell_type": "markdown",
41"metadata": {
42"id": "Wd2rYyussA8K"
43},
44"source": [
45"## Installing Haystack\n",
46"\n",
47"To start, let's install the latest release of Haystack with `pip`:"
48]
49},
50{
51"cell_type": "code",
52"execution_count": null,
53"metadata": {
54"colab": {
55"base_uri": "https://localhost:8080/"
56},
57"id": "5W4mAfAasA8L",
58"outputId": "5fd29b21-811d-499c-bbbc-9599f96e40d9"
59},
60"outputs": [],
61"source": [
62"%%bash\n",
63"\n",
64"pip install --upgrade pip\n",
65"pip install farm-haystack[colab,inference]"
66]
67},
68{
69"attachments": {},
70"cell_type": "markdown",
71"metadata": {
72"id": "6q-xTi6ksA8L"
73},
74"source": [
75"### Enabling Telemetry\n",
76"Knowing you're using this tutorial helps us decide where to invest our efforts to build a better product but you can always opt out by commenting the following line. See [Telemetry](https://docs.haystack.deepset.ai/docs/telemetry) for more details."
77]
78},
79{
80"cell_type": "code",
81"execution_count": null,
82"metadata": {
83"id": "QadbeTutsA8L"
84},
85"outputs": [],
86"source": [
87"from haystack.telemetry import tutorial_running\n",
88"\n",
89"tutorial_running(11)"
90]
91},
92{
93"attachments": {},
94"cell_type": "markdown",
95"metadata": {
96"collapsed": false,
97"id": "CwZDy9f-sA8M",
98"pycharm": {
99"name": "#%% md\n"
100}
101},
102"source": [
103"## Logging\n",
104"\n",
105"We configure how logging messages should be displayed and which log level should be used before importing Haystack.\n",
106"Example log message:\n",
107"INFO - haystack.utils.preprocessing - Converting data/tutorial1/218_Olenna_Tyrell.txt\n",
108"Default log level in basicConfig is WARNING so the explicit parameter is not necessary but can be changed easily:"
109]
110},
111{
112"cell_type": "code",
113"execution_count": null,
114"metadata": {
115"id": "1YXK3IqDsA8M",
116"pycharm": {
117"name": "#%%\n"
118}
119},
120"outputs": [],
121"source": [
122"import logging\n",
123"\n",
124"logging.basicConfig(format=\"%(levelname)s - %(name)s - %(message)s\", level=logging.WARNING)\n",
125"logging.getLogger(\"haystack\").setLevel(logging.INFO)"
126]
127},
128{
129"attachments": {},
130"cell_type": "markdown",
131"metadata": {
132"id": "zceM4-j_sA8M"
133},
134"source": [
135"## Initializing the DocumentStore\n",
136"\n",
137"A DocumentStore stores the Documents that a system uses to retrieve or find answers to your questions. In this tutorial, you'll use the `InMemoryDocumentStore`.\n",
138"\n",
139"Let's initialize the DocumentStore."
140]
141},
142{
143"cell_type": "code",
144"execution_count": null,
145"metadata": {
146"colab": {
147"base_uri": "https://localhost:8080/"
148},
149"id": "ktcvOryoCudy",
150"outputId": "09747c54-aae4-4e60-b309-380b676657e9"
151},
152"outputs": [],
153"source": [
154"from haystack.document_stores import InMemoryDocumentStore\n",
155"\n",
156"document_store = InMemoryDocumentStore(use_bm25=True)"
157]
158},
159{
160"attachments": {},
161"cell_type": "markdown",
162"metadata": {
163"collapsed": false,
164"id": "lDs0kKPmsA8N",
165"pycharm": {
166"name": "#%% md\n"
167}
168},
169"source": [
170"## Fetching and Writing Documents\n",
171"\n",
172"Let's fetch the txt files (in this case, pages from the Game of Thrones wiki) and prepare them so that they can be indexed into the DocumentStore:"
173]
174},
175{
176"cell_type": "code",
177"execution_count": null,
178"metadata": {},
179"outputs": [],
180"source": []
181},
182{
183"cell_type": "code",
184"execution_count": null,
185"metadata": {
186"id": "V80mCVopsA8N",
187"pycharm": {
188"name": "#%%\n"
189}
190},
191"outputs": [],
192"source": [
193"from haystack.utils import fetch_archive_from_http, convert_files_to_docs, clean_wiki_text\n",
194"\n",
195"# Download and prepare data - 517 Wikipedia articles for Game of Thrones\n",
196"doc_dir = \"data/tutorial11\"\n",
197"s3_url = \"https://s3.eu-central-1.amazonaws.com/deepset.ai-farm-qa/datasets/documents/wiki_gameofthrones_txt11.zip\"\n",
198"fetch_archive_from_http(url=s3_url, output_dir=doc_dir)\n",
199"\n",
200"# convert files to dicts containing documents that can be indexed to our datastore\n",
201"got_docs = convert_files_to_docs(dir_path=doc_dir, clean_func=clean_wiki_text, split_paragraphs=True)\n",
202"document_store.delete_documents()\n",
203"document_store.write_documents(got_docs)"
204]
205},
206{
207"cell_type": "code",
208"execution_count": null,
209"metadata": {},
210"outputs": [],
211"source": [
212"from haystack.nodes import BM25Retriever, EmbeddingRetriever, FARMReader\n",
213"\n",
214"bm25_retriever = BM25Retriever()\n",
215"\n",
216"from haystack.pipelines import DocumentSearchPipeline\n",
217"from haystack.utils import print_documents\n",
218"\n",
219"p_retrieval = DocumentSearchPipeline(bm25_retriever)\n",
220"# res = p_retrieval.run(query=\"Who is the father of Arya Stark?\", params={\"Retriever\": {\"top_k\": 10}})\n",
221"# print_documents(res, max_text_len=200)"
222]
223},
224{
225"attachments": {},
226"cell_type": "markdown",
227"metadata": {
228"collapsed": false,
229"id": "X79w6kHrsA8N",
230"pycharm": {
231"name": "#%% md\n"
232}
233},
234"source": [
235"## Initializing Core Components\n",
236"\n",
237"Here we initialize the core components that we will be gluing together using the `Pipeline` class.\n",
238"Initialize a `BM25Retriever`, an `EmbeddingRetriever`, and a `FARMReader`.\n",
239"You can combine these components to create a classic Retriever-Reader pipeline that is designed\n",
240"to perform Open Domain Question Answering."
241]
242},
243{
244"cell_type": "code",
245"execution_count": null,
246"metadata": {
247"id": "jJFkt1LVsA8N",
248"pycharm": {
249"name": "#%%\n"
250}
251},
252"outputs": [],
253"source": [
254"from haystack.nodes import BM25Retriever, EmbeddingRetriever, FARMReader\n",
255"\n",
256"# Initialize Sparse Retriever\n",
257"bm25_retriever = BM25Retriever(document_store=document_store)\n",
258"\n",
259"# Initialize embedding Retriever\n",
260"embedding_retriever = EmbeddingRetriever(\n",
261" document_store=document_store, embedding_model=\"sentence-transformers/multi-qa-mpnet-base-dot-v1\"\n",
262")\n",
263"document_store.update_embeddings(embedding_retriever, update_existing_embeddings=False)\n",
264"\n",
265"# Initialize Reader\n",
266"reader = FARMReader(model_name_or_path=\"deepset/roberta-base-squad2\")"
267]
268},
269{
270"attachments": {},
271"cell_type": "markdown",
272"metadata": {
273"collapsed": false,
274"id": "RwqtQRoAsA8N",
275"pycharm": {
276"name": "#%% md\n"
277}
278},
279"source": [
280"## Prebuilt Pipelines\n",
281"\n",
282"Haystack features many prebuilt pipelines that cover common tasks. The most used one is `ExtractiveQAPipeline`.\n",
283"Now, initialize the ExtractiveQAPipeline and run it with a query:"
284]
285},
286{
287"cell_type": "code",
288"execution_count": null,
289"metadata": {
290"colab": {
291"base_uri": "https://localhost:8080/",
292"height": 483,
293"referenced_widgets": [
294"3ff3b8f606f44adfa413314526d0f51b",
295"4b436abd81db41ad98f24f3148bb1fcb",
296"a20d8628bdb5486a8ccab95660a986c3",
297"42c2a9dbc747478e9f4691d01f1246da",
298"616af910d6ba45039b3b455631fe545c",
299"422ce6e179ca44439e0e1bb574daecc8",
300"5f05f9f76d5149e0a3c42e08dd85a82b",
301"4c514834225147eab4ba48b7ece93ecb",
302"c70f352bec2f441990a5e538cf644d6a",
303"4afe18f598764e90807ac2f32fca4853",
304"63850c8393aa4c1aa8a5e195857ceca3"
305]
306},
307"id": "fZZ_Tt08sA8N",
308"outputId": "7aa1446f-6de7-45df-cd4e-f3b333610294",
309"pycharm": {
310"name": "#%%\n"
311}
312},
313"outputs": [],
314"source": [
315"from haystack.pipelines import ExtractiveQAPipeline\n",
316"from haystack.utils import print_answers\n",
317"\n",
318"p_extractive_premade = ExtractiveQAPipeline(reader=reader, retriever=bm25_retriever)\n",
319"res = p_extractive_premade.run(\n",
320" query=\"Who is the father of Arya Stark?\", params={\"Retriever\": {\"top_k\": 10}, \"Reader\": {\"top_k\": 5}}\n",
321")\n",
322"print_answers(res, details=\"minimum\")"
323]
324},
325{
326"attachments": {},
327"cell_type": "markdown",
328"metadata": {
329"collapsed": false,
330"id": "PqKe8Nw9sA8N"
331},
332"source": [
333"If you want to just do the retrieval step, you can use a DocumentSearchPipeline:"
334]
335},
336{
337"cell_type": "code",
338"execution_count": null,
339"metadata": {
340"colab": {
341"base_uri": "https://localhost:8080/",
342"height": 1000,
343"referenced_widgets": [
344"7f57abc22d3a4231a6b02eb025b35f49",
345"a9e34231df9548e584c81ea89b814ee6",
346"9989d5d79a3a41fa9bc0431465997611",
347"f73977385dce49bead6c2012f2e42f71",
348"cc2586d0b77349b2b68a0b05e410f95f",
349"c78aa680ada54f29bc8bbe8ce94dfe31",
350"0e3b35c207e34b738c179ed6de3fb906",
351"333a2e1b38f14049bcd8633fb614dfd1",
352"05e19ba65d5b4df09c6a93551affbf67",
353"bd2fb1c05642482bbed1e4f4e2824f80",
354"5b64bdd8ce404f8290511d9bf9c7f29f"
355]
356},
357"id": "lA1DyIhLsA8N",
358"outputId": "018fa621-f08e-424c-ef4a-8d7f50231ec0",
359"pycharm": {
360"name": "#%%\n"
361}
362},
363"outputs": [],
364"source": [
365"from haystack.pipelines import DocumentSearchPipeline\n",
366"from haystack.utils import print_documents\n",
367"\n",
368"p_retrieval = DocumentSearchPipeline(embedding_retriever)\n",
369"res = p_retrieval.run(query=\"Who is the father of Arya Stark?\", params={\"Retriever\": {\"top_k\": 10}})\n",
370"print_documents(res, max_text_len=200)"
371]
372},
373{
374"attachments": {},
375"cell_type": "markdown",
376"metadata": {
377"collapsed": false,
378"id": "_ISxlB1zsA8N"
379},
380"source": [
381"Haystack features prebuilt pipelines to do:\n",
382"- document search (DocumentSearchPipeline),\n",
383"- document search with summarization (SearchSummarizationPipeline)\n",
384"- FAQ style QA (FAQPipeline)\n",
385"- translated search (TranslationWrapperPipeline)\n",
386"To find out more about these pipelines, have a look at our [documentation](https://docs.haystack.deepset.ai/docs/pipelines).\n"
387]
388},
389{
390"attachments": {},
391"cell_type": "markdown",
392"metadata": {
393"collapsed": false,
394"id": "YYLCqoYTsA8O",
395"pycharm": {
396"name": "#%% md\n"
397}
398},
399"source": [
400"## Generating a Pipeline Diagram\n",
401"\n",
402"With any Pipeline, whether prebuilt or custom constructed,\n",
403"you can save a diagram showing how all the components are connected.\n",
404"\n",
405"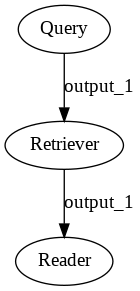"
406]
407},
408{
409"cell_type": "code",
410"execution_count": null,
411"metadata": {
412"colab": {
413"base_uri": "https://localhost:8080/"
414},
415"id": "krDbpxY3sA8O",
416"outputId": "fcd13061-591b-451d-eb05-f93f3546b119",
417"pycharm": {
418"name": "#%%\n"
419}
420},
421"outputs": [],
422"source": [
423"# Uncomment the following to generate the images\n",
424"# !apt install libgraphviz-dev\n",
425"# !pip install pygraphviz\n",
426"\n",
427"# p_extractive_premade.draw(\"pipeline_extractive_premade.png\")\n",
428"# p_retrieval.draw(\"pipeline_retrieval.png\")"
429]
430},
431{
432"attachments": {},
433"cell_type": "markdown",
434"metadata": {
435"collapsed": false,
436"id": "RB9PEOKssA8O",
437"pycharm": {
438"name": "#%% md\n"
439}
440},
441"source": [
442"## Custom Extractive QA Pipeline\n",
443"\n",
444"If you need a custom pipeline for your task or add extra nodes to a pipeline, you can create a pipeline from scratch instead of using a prebuilt one.\n",
445"\n",
446"Now, try to rebuild the ExtractiveQAPipeline using the generic Pipeline class.\n",
447"You can do this by adding the building blocks as shown in the graph."
448]
449},
450{
451"cell_type": "code",
452"execution_count": null,
453"metadata": {
454"colab": {
455"base_uri": "https://localhost:8080/",
456"height": 864,
457"referenced_widgets": [
458"579ff5067d6b46c893612bd233906b34",
459"9a4a31fda4e64a27ae2c884ad1e9d9c9",
460"3ee68111e3844c70a6ea72f295ef0392",
461"d5c66b0a453f4a03954fabfd85187e83",
462"650915e12d2644c38cf2f6ec72faba92",
463"ac6c13dab196486d87d249b3f5382e86",
464"d5e111a594d3475dbdff20071822e139",
465"93a607e6574f4a0fb3b9b1463a4aad4c",
466"8c4d1d61155e4064bdf76599be6c8bbe",
467"99945c16563d40a5aa0825a1134a6300",
468"f39c9f27960e4ff99bd7aef02f14ffd2"
469]
470},
471"id": "x5WMGbCgsA8O",
472"outputId": "a22deeba-b303-43cf-b51f-4b1514c5d85a",
473"pycharm": {
474"name": "#%%\n"
475}
476},
477"outputs": [],
478"source": [
479"from haystack.pipelines import Pipeline\n",
480"\n",
481"# Custom built extractive QA pipeline\n",
482"p_extractive = Pipeline()\n",
483"p_extractive.add_node(component=bm25_retriever, name=\"Retriever\", inputs=[\"Query\"])\n",
484"p_extractive.add_node(component=reader, name=\"Reader\", inputs=[\"Retriever\"])\n",
485"\n",
486"# Now we can run it\n",
487"res = p_extractive.run(query=\"Who is the father of Arya Stark?\", params={\"Retriever\": {\"top_k\": 10}})\n",
488"print_answers(res, details=\"minimum\")\n",
489"\n",
490"# Uncomment the following to generate the pipeline image\n",
491"# p_extractive.draw(\"pipeline_extractive.png\")"
492]
493},
494{
495"attachments": {},
496"cell_type": "markdown",
497"metadata": {
498"collapsed": false,
499"id": "Z_R1488-sA8O",
500"pycharm": {
501"name": "#%% md\n"
502}
503},
504"source": [
505"## Combining Retrievers Using a Custom Pipeline\n",
506"\n",
507"Pipelines offer a very simple way to ensemble together different components.\n",
508"In this example, you'll combine the power of an `EmbeddingRetriever`\n",
509"with the keyword based `BM25Retriever`.\n",
510"See our [documentation](https://docs.haystack.deepset.ai/docs/retriever#deeper-dive-vector-based-vs-keyword-based) to understand why\n",
511"you might want to combine a dense and sparse retriever.\n",
512"\n",
513"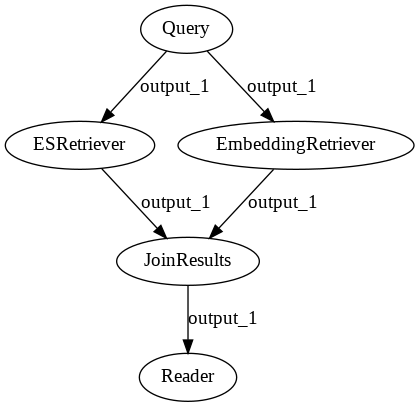\n",
514"\n",
515"Here you'll use a `JoinDocuments` node to merge the predictions from each retriever:"
516]
517},
518{
519"cell_type": "code",
520"execution_count": null,
521"metadata": {
522"colab": {
523"base_uri": "https://localhost:8080/",
524"height": 862,
525"referenced_widgets": [
526"8e01cbb665f442d494afc844eb4c1eac",
527"68b55859ea7b420a9bd917b85d122864",
528"c88f10e17ab94da3852ae0f8ac7ff7db",
529"3ef8a6849b23425ba3074c6353c58f83",
530"c7673d0c64df414981cfc7246a0e01e6",
531"9e52e775dc5640be920eebc454aaa67c",
532"8f3b6fb4b42f4382a6de2017fb79f5b8",
533"eaa5a7cf338f4c4faf1f4221684f6fa1",
534"2d800606643942e0b0616c1655142793",
535"035f6e1399144c2a8cb45b8b5be92f32",
536"dbd48e8c1a9445e3b757609cf0b60fa3",
537"3904531861a44de996c9a48170d06f7e",
538"0253c9956d434314bc7903c5b0123530",
539"39c38fff58154f8291a4635387230036",
540"c7d62339c034489b8dd9ade762892ff8",
541"dbb57469064a479085473de8e71ce4d0",
542"897b099603c240f096b40b8da22f8aaa",
543"5f537475318e43e4b3b7e54b43a978b5",
544"c050d37c2f4642b0925abd9e2eb06d99",
545"a1022f00dda143778c1a1ebc80f7e878",
546"d861455dc8344b1fbb03c966e8a803ad",
547"156b4ec959084fbdbcdfb9f7a12ece5f"
548]
549},
550"id": "jHY1iwlysA8O",
551"outputId": "2c278d74-0de9-452d-e7d8-539930a278e9",
552"pycharm": {
553"name": "#%%\n"
554}
555},
556"outputs": [],
557"source": [
558"from haystack.nodes import JoinDocuments\n",
559"\n",
560"# Create ensembled pipeline\n",
561"p_ensemble = Pipeline()\n",
562"p_ensemble.add_node(component=bm25_retriever, name=\"BM25Retriever\", inputs=[\"Query\"])\n",
563"p_ensemble.add_node(component=embedding_retriever, name=\"EmbeddingRetriever\", inputs=[\"Query\"])\n",
564"p_ensemble.add_node(\n",
565" component=JoinDocuments(join_mode=\"concatenate\"), name=\"JoinResults\", inputs=[\"BM25Retriever\", \"EmbeddingRetriever\"]\n",
566")\n",
567"p_ensemble.add_node(component=reader, name=\"Reader\", inputs=[\"JoinResults\"])\n",
568"\n",
569"# Uncomment the following to generate the pipeline image\n",
570"# p_ensemble.draw(\"pipeline_ensemble.png\")\n",
571"\n",
572"# Run pipeline\n",
573"res = p_ensemble.run(\n",
574" query=\"Who is the father of Arya Stark?\", params={\"EmbeddingRetriever\": {\"top_k\": 5}, \"BM25Retriever\": {\"top_k\": 5}}\n",
575")\n",
576"print_answers(res, details=\"minimum\")"
577]
578},
579{
580"attachments": {},
581"cell_type": "markdown",
582"metadata": {
583"collapsed": false,
584"id": "Z2_Sonx6sA8O",
585"pycharm": {
586"name": "#%% md\n"
587}
588},
589"source": [
590"## Custom Nodes\n",
591"\n",
592"Nodes are relatively simple objects\n",
593"and we encourage our users to design their own if they don't see one that fits their use case\n",
594"\n",
595"The only requirements are:\n",
596"- Create a class that inherits `BaseComponent`.\n",
597"- Add a method run() to your class. Add the mandatory and optional arguments it needs to process. These arguments must be passed as input to the pipeline, inside `params`, or output by preceding nodes.\n",
598"- Add processing logic inside the run() (e.g. reformatting the query).\n",
599"- Return a tuple that contains your output data (for the next node)\n",
600"and the name of the outgoing edge (by default \"output_1\" for nodes that have one output)\n",
601"- Add a class attribute outgoing_edges = 1 that defines the number of output options from your node. You only need a higher number here if you have a decision node (see below).\n",
602"\n",
603"Here is a template for a custom node:"
604]
605},
606{
607"cell_type": "code",
608"execution_count": null,
609"metadata": {
610"id": "1cZYUg4hsA8O",
611"pycharm": {
612"name": "#%%\n"
613}
614},
615"outputs": [],
616"source": [
617"from haystack import BaseComponent\n",
618"from typing import Optional, List\n",
619"\n",
620"\n",
621"class CustomNode(BaseComponent):\n",
622" outgoing_edges = 1\n",
623"\n",
624" def run(self, query: str, my_optional_param: Optional[int]):\n",
625" # process the inputs\n",
626" output = {\"my_output\": ...}\n",
627" return output, \"output_1\"\n",
628"\n",
629" def run_batch(self, queries: List[str], my_optional_param: Optional[int]):\n",
630" # process the inputs\n",
631" output = {\"my_output\": ...}\n",
632" return output, \"output_1\""
633]
634},
635{
636"attachments": {},
637"cell_type": "markdown",
638"metadata": {
639"collapsed": false,
640"id": "810qrYXwsA8O",
641"pycharm": {
642"name": "#%% md\n"
643}
644},
645"source": [
646"## Decision Nodes\n",
647"\n",
648"Decision Nodes help you route your data so that only certain branches of your `Pipeline` are run.\n",
649"One popular use case for such query classifiers is routing keyword queries to Elasticsearch and questions to EmbeddingRetriever + Reader.\n",
650"With this approach you keep optimal speed and simplicity for keywords while going deep with transformers when it's most helpful.\n",
651"\n",
652"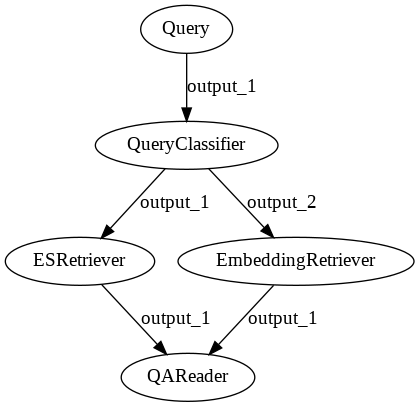\n",
653"\n",
654"Though this looks very similar to the ensembled pipeline shown above,\n",
655"the key difference is that only one of the retrievers is run for each request.\n",
656"By contrast both retrievers are always run in the ensembled approach.\n",
657"\n",
658"Below, we define a very naive `QueryClassifier` and show how to use it:"
659]
660},
661{
662"cell_type": "code",
663"execution_count": null,
664"metadata": {
665"colab": {
666"base_uri": "https://localhost:8080/",
667"height": 619,
668"referenced_widgets": [
669"85133ad2451b4f1db002b6b49a01f2d2",
670"6dcbaa9a020048119de893c5dd3fe5b3",
671"9d4376e02eb7424ba13297548867ac44",
672"353d4c638c3b4ac2b6bd0411fd6b1dca",
673"054fa49e5ff8468eb24c38666a9783aa",
674"5f8dea9534fd47e9a1efddecc4e6da3d",
675"33c13d2da30c47b18c77a1cef006497d",
676"170f6a48eb4249e3a44d56878415d94f",
677"55405182fbc24485934754b53eac871b",
678"ad54965c74384ab283e7c638d254d763",
679"ef2447b1e70d499ebc080c4e3b46f35c",
680"15e92e595d5345959218cccec3c4c550",
681"ca94bc5b7543455ebe388c4c934b7664",
682"7091ed26beba439690f78dfa715542c5",
683"936281119c65404f8af72d03b48a23a4",
684"ce171e8d143d48569f426f3870ea977a",
685"ef3fd3ff0c1342958346746f939aa48a",
686"dfe261365e144254ae00ea321579b860",
687"c72dbeb5557b4ac7bc46c957bab2485f",
688"d8f756ab4c9245709c40a48df7842c07",
689"5c01678b9ac64366b728da1cecd4e1c0",
690"6ee5d390d87b48888029b917805ac640",
691"fd2f080261c74992bd7fb9a0b6f6010b",
692"7fd49ced837540d2b3e560a6dd507ac3",
693"7f69e47ac44c401f9b26f8c88de4c481",
694"0466c5b6e97b467b844ff2b72ab9de8e",
695"6baf2644608242a6a431cd3939201d51",
696"c0cd6e5f853b4ee0858d3b4c53a102ee",
697"77007e83bee849deb05fbfd2b9321310",
698"be6dd7e2f43748d490deda9bab833ce4",
699"8cdc612b706e4d0b8a2528d3176b575a",
700"d5630f9ff5f546c9871f5f2b76fb305a",
701"b096be01767543418953eae9bc148cb1"
702]
703},
704"id": "tzHCRC0ksA8O",
705"outputId": "b0fffbf8-f7dd-4cba-f3f3-fa91b6a24ed0",
706"pycharm": {
707"name": "#%%\n"
708}
709},
710"outputs": [],
711"source": [
712"class CustomQueryClassifier(BaseComponent):\n",
713" outgoing_edges = 2\n",
714"\n",
715" def run(self, query: str):\n",
716" if \"?\" in query:\n",
717" return {}, \"output_2\"\n",
718" else:\n",
719" return {}, \"output_1\"\n",
720"\n",
721" def run_batch(self, queries: List[str]):\n",
722" split = {\"output_1\": {\"queries\": []}, \"output_2\": {\"queries\": []}}\n",
723" for query in queries:\n",
724" if \"?\" in query:\n",
725" split[\"output_2\"][\"queries\"].append(query)\n",
726" else:\n",
727" split[\"output_1\"][\"queries\"].append(query)\n",
728"\n",
729" return split, \"split\"\n",
730"\n",
731"\n",
732"# Here we build the pipeline\n",
733"p_classifier = Pipeline()\n",
734"p_classifier.add_node(component=CustomQueryClassifier(), name=\"QueryClassifier\", inputs=[\"Query\"])\n",
735"p_classifier.add_node(component=bm25_retriever, name=\"BM25Retriever\", inputs=[\"QueryClassifier.output_1\"])\n",
736"p_classifier.add_node(component=embedding_retriever, name=\"EmbeddingRetriever\", inputs=[\"QueryClassifier.output_2\"])\n",
737"p_classifier.add_node(component=reader, name=\"QAReader\", inputs=[\"BM25Retriever\", \"EmbeddingRetriever\"])\n",
738"# Uncomment the following to generate the pipeline image\n",
739"# p_classifier.draw(\"pipeline_classifier.png\")\n",
740"\n",
741"# Run only the dense retriever on the full sentence query\n",
742"res_1 = p_classifier.run(query=\"Who is the father of Arya Stark?\")\n",
743"print(\"Embedding Retriever Results\" + \"\\n\" + \"=\" * 15)\n",
744"print_answers(res_1)\n",
745"\n",
746"# Run only the sparse retriever on a keyword based query\n",
747"res_2 = p_classifier.run(query=\"Arya Stark father\")\n",
748"print(\"BM25Retriever Results\" + \"\\n\" + \"=\" * 15)\n",
749"print_answers(res_2)"
750]
751},
752{
753"attachments": {},
754"cell_type": "markdown",
755"metadata": {
756"collapsed": false,
757"id": "KhsZ_vZqsA8O",
758"pycharm": {
759"name": "#%% md\n"
760}
761},
762"source": [
763"## Evaluation Nodes\n",
764"\n",
765"We have also designed a set of nodes that can be used to evaluate the performance of a system.\n",
766"Have a look at our [tutorial](https://haystack.deepset.ai/tutorials/05_evaluation) to get hands on with the code and learn more about Evaluation Nodes!"
767]
768},
769{
770"attachments": {},
771"cell_type": "markdown",
772"metadata": {
773"collapsed": false,
774"id": "D7d-L6A3sA8O",
775"pycharm": {
776"name": "#%% md\n"
777}
778},
779"source": [
780"## Debugging Pipelines\n",
781"\n",
782"You can print out debug information from nodes in your pipelines in a few different ways."
783]
784},
785{
786"cell_type": "code",
787"execution_count": null,
788"metadata": {
789"colab": {
790"base_uri": "https://localhost:8080/",
791"height": 1000,
792"referenced_widgets": [
793"ec9801b7916d4911be6cd6c806642d4a",
794"4402e159d59a4518a894d7ded2025b21",
795"114859f36d21473e8fb44f4c27a71ca3",
796"3fa2749682e84da5b073250e391e9970",
797"bbfb9e1799aa4580aef7cbf88d604d84",
798"c4539733aed34c7b940de5da7edfa82a",
799"2a0ebf357d0f4ed3b283b561e5ab5774",
800"c0b9068361404c5ab60bb7d4b3dca265",
801"a0d604c26d75411b870a729695ea8341",
802"4447f0b788e944c184ca8b885a105d61",
803"e530c06891274d41b5d8a2b20e160ee4",
804"bed20d405095472e8b5166f1d9d1b041",
805"a53cdfb5315a42beb72569956916cdfa",
806"f12d6748a4bc478c92d8e8ed8730cce2",
807"b20df6374f924e7e896d58e93fd45ae4",
808"8780553fdded4f0b906e0ce670a1657e",
809"96e57e14ae6e45bab18b8f940c048507",
810"f6bac06384a84aea8a470bf612e90d2a",
811"7165f68ae5fd4b57a3f30942519f2d29",
812"4c41e83814e44a578a6e62585cafd34d",
813"9258bf70f05f4f3d96621a5c5a2a8a75",
814"38e8dfef6cbf4f2b82471b74d3814177",
815"e19b0f2f1eaf4d58b25e59deee4cc70c",
816"7ee82a73e82c4ad29a15bf336095fa49",
817"ddb287b8aa9c40fbb4bc38b84c2f54d5",
818"9962218c39cf4d20abc2460278fd6808",
819"ed3a7ced6e0247ef93cd20fe3cc62591",
820"812a04255c8648158e4f82665ff2efce",
821"f54f6e8998f54d5c8d367d244158c261",
822"33b5df6b101a4b16bcd0fbb13c8980d9",
823"cf10491e88374c679c94b757ef207144",
824"633687b1bf3e49b2bbe5c8fcdf21b187",
825"9eb4bff064f7491d8e90bb7ab270d0c2",
826"f76772d14e4a4f0487044dcbd253f82e",
827"c321ff97706d465193a2b3dfcb107c1c",
828"842019f6aed44d41976b11a0352d4596",
829"729a5070b09f4b2aa511d58e2b5833d5",
830"8fb5aba463ac40a18063b4fc7cd3d879",
831"1816d2ee14014fe7b76402ceac0cfc67",
832"3298a7b7d5d14f198695f0769e527b60",
833"d3d1971a3e3748c9ac7483faafbee1a0",
834"25046a95109d4d25a2dd7bb70201c66d",
835"429276694dba48d2a5958f4945ede4b7",
836"95b8b7dcd2ff4152b8a684e5cb878a91"
837]
838},
839"id": "ZakI7jn9sA8O",
840"outputId": "93a8faf2-89ea-4190-f738-7648dfbd88cd",
841"pycharm": {
842"name": "#%%\n"
843}
844},
845"outputs": [],
846"source": [
847"# 1) You can set the `debug` attribute of a given node.\n",
848"bm25_retriever.debug = True\n",
849"\n",
850"# 2) You can provide `debug` as a parameter when running your pipeline\n",
851"result = p_classifier.run(query=\"Who is the father of Arya Stark?\", params={\"BM25Retriever\": {\"debug\": True}})\n",
852"\n",
853"# 3) You can provide the `debug` paramter to all nodes in your pipeline\n",
854"result = p_classifier.run(query=\"Who is the father of Arya Stark?\", params={\"debug\": True})\n",
855"\n",
856"result[\"_debug\"]"
857]
858},
859{
860"attachments": {},
861"cell_type": "markdown",
862"metadata": {
863"collapsed": false,
864"id": "VSTHlO55sA8S",
865"pycharm": {
866"name": "#%% md\n"
867}
868},
869"source": [
870"## Conclusion\n",
871"\n",
872"The possibilities are endless with the `Pipeline` class and we hope that this tutorial will inspire you\n",
873"to build custom pipelines that really work for your use case!"
874]
875}
876],
877"metadata": {
878"accelerator": "GPU",
879"colab": {
880"gpuType": "T4",
881"provenance": []
882},
883"kernelspec": {
884"display_name": "Python 3",
885"name": "python3"
886},
887"language_info": {
888"codemirror_mode": {
889"name": "ipython",
890"version": 3
891},
892"file_extension": ".py",
893"mimetype": "text/x-python",
894"name": "python",
895"nbconvert_exporter": "python",
896"pygments_lexer": "ipython3",
897"version": "3.9.12"
898},
899"vscode": {
900"interpreter": {
901"hash": "31f2aee4e71d21fbe5cf8b01ff0e069b9275f58929596ceb00d14d90e3e16cd6"
902}
903},
904"widgets": {
905"application/vnd.jupyter.widget-state+json": {
906"0253c9956d434314bc7903c5b0123530": {
907"model_module": "@jupyter-widgets/controls",
908"model_module_version": "1.5.0",
909"model_name": "HTMLModel",
910"state": {
911"_dom_classes": [],
912"_model_module": "@jupyter-widgets/controls",
913"_model_module_version": "1.5.0",
914"_model_name": "HTMLModel",
915"_view_count": null,
916"_view_module": "@jupyter-widgets/controls",
917"_view_module_version": "1.5.0",
918"_view_name": "HTMLView",
919"description": "",
920"description_tooltip": null,
921"layout": "IPY_MODEL_897b099603c240f096b40b8da22f8aaa",
922"placeholder": "",
923"style": "IPY_MODEL_5f537475318e43e4b3b7e54b43a978b5",
924"value": "Inferencing Samples: 100%"
925}
926},
927"035f6e1399144c2a8cb45b8b5be92f32": {
928"model_module": "@jupyter-widgets/base",
929"model_module_version": "1.2.0",
930"model_name": "LayoutModel",
931"state": {
932"_model_module": "@jupyter-widgets/base",
933"_model_module_version": "1.2.0",
934"_model_name": "LayoutModel",
935"_view_count": null,
936"_view_module": "@jupyter-widgets/base",
937"_view_module_version": "1.2.0",
938"_view_name": "LayoutView",
939"align_content": null,
940"align_items": null,
941"align_self": null,
942"border": null,
943"bottom": null,
944"display": null,
945"flex": null,
946"flex_flow": null,
947"grid_area": null,
948"grid_auto_columns": null,
949"grid_auto_flow": null,
950"grid_auto_rows": null,
951"grid_column": null,
952"grid_gap": null,
953"grid_row": null,
954"grid_template_areas": null,
955"grid_template_columns": null,
956"grid_template_rows": null,
957"height": null,
958"justify_content": null,
959"justify_items": null,
960"left": null,
961"margin": null,
962"max_height": null,
963"max_width": null,
964"min_height": null,
965"min_width": null,
966"object_fit": null,
967"object_position": null,
968"order": null,
969"overflow": null,
970"overflow_x": null,
971"overflow_y": null,
972"padding": null,
973"right": null,
974"top": null,
975"visibility": null,
976"width": null
977}
978},
979"0466c5b6e97b467b844ff2b72ab9de8e": {
980"model_module": "@jupyter-widgets/controls",
981"model_module_version": "1.5.0",
982"model_name": "HTMLModel",
983"state": {
984"_dom_classes": [],
985"_model_module": "@jupyter-widgets/controls",
986"_model_module_version": "1.5.0",
987"_model_name": "HTMLModel",
988"_view_count": null,
989"_view_module": "@jupyter-widgets/controls",
990"_view_module_version": "1.5.0",
991"_view_name": "HTMLView",
992"description": "",
993"description_tooltip": null,
994"layout": "IPY_MODEL_d5630f9ff5f546c9871f5f2b76fb305a",
995"placeholder": "",
996"style": "IPY_MODEL_b096be01767543418953eae9bc148cb1",
997"value": " 1/1 [00:00<00:00, 4.24 Batches/s]"
998}
999},
1000"054fa49e5ff8468eb24c38666a9783aa": {
1001"model_module": "@jupyter-widgets/base",
1002"model_module_version": "1.2.0",
1003"model_name": "LayoutModel",
1004"state": {
1005"_model_module": "@jupyter-widgets/base",
1006"_model_module_version": "1.2.0",
1007"_model_name": "LayoutModel",
1008"_view_count": null,
1009"_view_module": "@jupyter-widgets/base",
1010"_view_module_version": "1.2.0",
1011"_view_name": "LayoutView",
1012"align_content": null,
1013"align_items": null,
1014"align_self": null,
1015"border": null,
1016"bottom": null,
1017"display": null,
1018"flex": null,
1019"flex_flow": null,
1020"grid_area": null,
1021"grid_auto_columns": null,
1022"grid_auto_flow": null,
1023"grid_auto_rows": null,
1024"grid_column": null,
1025"grid_gap": null,
1026"grid_row": null,
1027"grid_template_areas": null,
1028"grid_template_columns": null,
1029"grid_template_rows": null,
1030"height": null,
1031"justify_content": null,
1032"justify_items": null,
1033"left": null,
1034"margin": null,
1035"max_height": null,
1036"max_width": null,
1037"min_height": null,
1038"min_width": null,
1039"object_fit": null,
1040"object_position": null,
1041"order": null,
1042"overflow": null,
1043"overflow_x": null,
1044"overflow_y": null,
1045"padding": null,
1046"right": null,
1047"top": null,
1048"visibility": null,
1049"width": null
1050}
1051},
1052"05e19ba65d5b4df09c6a93551affbf67": {
1053"model_module": "@jupyter-widgets/controls",
1054"model_module_version": "1.5.0",
1055"model_name": "ProgressStyleModel",
1056"state": {
1057"_model_module": "@jupyter-widgets/controls",
1058"_model_module_version": "1.5.0",
1059"_model_name": "ProgressStyleModel",
1060"_view_count": null,
1061"_view_module": "@jupyter-widgets/base",
1062"_view_module_version": "1.2.0",
1063"_view_name": "StyleView",
1064"bar_color": null,
1065"description_width": ""
1066}
1067},
1068"0e3b35c207e34b738c179ed6de3fb906": {
1069"model_module": "@jupyter-widgets/controls",
1070"model_module_version": "1.5.0",
1071"model_name": "DescriptionStyleModel",
1072"state": {
1073"_model_module": "@jupyter-widgets/controls",
1074"_model_module_version": "1.5.0",
1075"_model_name": "DescriptionStyleModel",
1076"_view_count": null,
1077"_view_module": "@jupyter-widgets/base",
1078"_view_module_version": "1.2.0",
1079"_view_name": "StyleView",
1080"description_width": ""
1081}
1082},
1083"114859f36d21473e8fb44f4c27a71ca3": {
1084"model_module": "@jupyter-widgets/controls",
1085"model_module_version": "1.5.0",
1086"model_name": "FloatProgressModel",
1087"state": {
1088"_dom_classes": [],
1089"_model_module": "@jupyter-widgets/controls",
1090"_model_module_version": "1.5.0",
1091"_model_name": "FloatProgressModel",
1092"_view_count": null,
1093"_view_module": "@jupyter-widgets/controls",
1094"_view_module_version": "1.5.0",
1095"_view_name": "ProgressView",
1096"bar_style": "success",
1097"description": "",
1098"description_tooltip": null,
1099"layout": "IPY_MODEL_c0b9068361404c5ab60bb7d4b3dca265",
1100"max": 1,
1101"min": 0,
1102"orientation": "horizontal",
1103"style": "IPY_MODEL_a0d604c26d75411b870a729695ea8341",
1104"value": 1
1105}
1106},
1107"156b4ec959084fbdbcdfb9f7a12ece5f": {
1108"model_module": "@jupyter-widgets/controls",
1109"model_module_version": "1.5.0",
1110"model_name": "DescriptionStyleModel",
1111"state": {
1112"_model_module": "@jupyter-widgets/controls",
1113"_model_module_version": "1.5.0",
1114"_model_name": "DescriptionStyleModel",
1115"_view_count": null,
1116"_view_module": "@jupyter-widgets/base",
1117"_view_module_version": "1.2.0",
1118"_view_name": "StyleView",
1119"description_width": ""
1120}
1121},
1122"15e92e595d5345959218cccec3c4c550": {
1123"model_module": "@jupyter-widgets/controls",
1124"model_module_version": "1.5.0",
1125"model_name": "HBoxModel",
1126"state": {
1127"_dom_classes": [],
1128"_model_module": "@jupyter-widgets/controls",
1129"_model_module_version": "1.5.0",
1130"_model_name": "HBoxModel",
1131"_view_count": null,
1132"_view_module": "@jupyter-widgets/controls",
1133"_view_module_version": "1.5.0",
1134"_view_name": "HBoxView",
1135"box_style": "",
1136"children": [
1137"IPY_MODEL_ca94bc5b7543455ebe388c4c934b7664",
1138"IPY_MODEL_7091ed26beba439690f78dfa715542c5",
1139"IPY_MODEL_936281119c65404f8af72d03b48a23a4"
1140],
1141"layout": "IPY_MODEL_ce171e8d143d48569f426f3870ea977a"
1142}
1143},
1144"170f6a48eb4249e3a44d56878415d94f": {
1145"model_module": "@jupyter-widgets/base",
1146"model_module_version": "1.2.0",
1147"model_name": "LayoutModel",
1148"state": {
1149"_model_module": "@jupyter-widgets/base",
1150"_model_module_version": "1.2.0",
1151"_model_name": "LayoutModel",
1152"_view_count": null,
1153"_view_module": "@jupyter-widgets/base",
1154"_view_module_version": "1.2.0",
1155"_view_name": "LayoutView",
1156"align_content": null,
1157"align_items": null,
1158"align_self": null,
1159"border": null,
1160"bottom": null,
1161"display": null,
1162"flex": null,
1163"flex_flow": null,
1164"grid_area": null,
1165"grid_auto_columns": null,
1166"grid_auto_flow": null,
1167"grid_auto_rows": null,
1168"grid_column": null,
1169"grid_gap": null,
1170"grid_row": null,
1171"grid_template_areas": null,
1172"grid_template_columns": null,
1173"grid_template_rows": null,
1174"height": null,
1175"justify_content": null,
1176"justify_items": null,
1177"left": null,
1178"margin": null,
1179"max_height": null,
1180"max_width": null,
1181"min_height": null,
1182"min_width": null,
1183"object_fit": null,
1184"object_position": null,
1185"order": null,
1186"overflow": null,
1187"overflow_x": null,
1188"overflow_y": null,
1189"padding": null,
1190"right": null,
1191"top": null,
1192"visibility": null,
1193"width": null
1194}
1195},
1196"1816d2ee14014fe7b76402ceac0cfc67": {
1197"model_module": "@jupyter-widgets/base",
1198"model_module_version": "1.2.0",
1199"model_name": "LayoutModel",
1200"state": {
1201"_model_module": "@jupyter-widgets/base",
1202"_model_module_version": "1.2.0",
1203"_model_name": "LayoutModel",
1204"_view_count": null,
1205"_view_module": "@jupyter-widgets/base",
1206"_view_module_version": "1.2.0",
1207"_view_name": "LayoutView",
1208"align_content": null,
1209"align_items": null,
1210"align_self": null,
1211"border": null,
1212"bottom": null,
1213"display": null,
1214"flex": null,
1215"flex_flow": null,
1216"grid_area": null,
1217"grid_auto_columns": null,
1218"grid_auto_flow": null,
1219"grid_auto_rows": null,
1220"grid_column": null,
1221"grid_gap": null,
1222"grid_row": null,
1223"grid_template_areas": null,
1224"grid_template_columns": null,
1225"grid_template_rows": null,
1226"height": null,
1227"justify_content": null,
1228"justify_items": null,
1229"left": null,
1230"margin": null,
1231"max_height": null,
1232"max_width": null,
1233"min_height": null,
1234"min_width": null,
1235"object_fit": null,
1236"object_position": null,
1237"order": null,
1238"overflow": null,
1239"overflow_x": null,
1240"overflow_y": null,
1241"padding": null,
1242"right": null,
1243"top": null,
1244"visibility": null,
1245"width": null
1246}
1247},
1248"25046a95109d4d25a2dd7bb70201c66d": {
1249"model_module": "@jupyter-widgets/controls",
1250"model_module_version": "1.5.0",
1251"model_name": "ProgressStyleModel",
1252"state": {
1253"_model_module": "@jupyter-widgets/controls",
1254"_model_module_version": "1.5.0",
1255"_model_name": "ProgressStyleModel",
1256"_view_count": null,
1257"_view_module": "@jupyter-widgets/base",
1258"_view_module_version": "1.2.0",
1259"_view_name": "StyleView",
1260"bar_color": null,
1261"description_width": ""
1262}
1263},
1264"2a0ebf357d0f4ed3b283b561e5ab5774": {
1265"model_module": "@jupyter-widgets/controls",
1266"model_module_version": "1.5.0",
1267"model_name": "DescriptionStyleModel",
1268"state": {
1269"_model_module": "@jupyter-widgets/controls",
1270"_model_module_version": "1.5.0",
1271"_model_name": "DescriptionStyleModel",
1272"_view_count": null,
1273"_view_module": "@jupyter-widgets/base",
1274"_view_module_version": "1.2.0",
1275"_view_name": "StyleView",
1276"description_width": ""
1277}
1278},
1279"2d800606643942e0b0616c1655142793": {
1280"model_module": "@jupyter-widgets/controls",
1281"model_module_version": "1.5.0",
1282"model_name": "ProgressStyleModel",
1283"state": {
1284"_model_module": "@jupyter-widgets/controls",
1285"_model_module_version": "1.5.0",
1286"_model_name": "ProgressStyleModel",
1287"_view_count": null,
1288"_view_module": "@jupyter-widgets/base",
1289"_view_module_version": "1.2.0",
1290"_view_name": "StyleView",
1291"bar_color": null,
1292"description_width": ""
1293}
1294},
1295"3298a7b7d5d14f198695f0769e527b60": {
1296"model_module": "@jupyter-widgets/controls",
1297"model_module_version": "1.5.0",
1298"model_name": "DescriptionStyleModel",
1299"state": {
1300"_model_module": "@jupyter-widgets/controls",
1301"_model_module_version": "1.5.0",
1302"_model_name": "DescriptionStyleModel",
1303"_view_count": null,
1304"_view_module": "@jupyter-widgets/base",
1305"_view_module_version": "1.2.0",
1306"_view_name": "StyleView",
1307"description_width": ""
1308}
1309},
1310"333a2e1b38f14049bcd8633fb614dfd1": {
1311"model_module": "@jupyter-widgets/base",
1312"model_module_version": "1.2.0",
1313"model_name": "LayoutModel",
1314"state": {
1315"_model_module": "@jupyter-widgets/base",
1316"_model_module_version": "1.2.0",
1317"_model_name": "LayoutModel",
1318"_view_count": null,
1319"_view_module": "@jupyter-widgets/base",
1320"_view_module_version": "1.2.0",
1321"_view_name": "LayoutView",
1322"align_content": null,
1323"align_items": null,
1324"align_self": null,
1325"border": null,
1326"bottom": null,
1327"display": null,
1328"flex": null,
1329"flex_flow": null,
1330"grid_area": null,
1331"grid_auto_columns": null,
1332"grid_auto_flow": null,
1333"grid_auto_rows": null,
1334"grid_column": null,
1335"grid_gap": null,
1336"grid_row": null,
1337"grid_template_areas": null,
1338"grid_template_columns": null,
1339"grid_template_rows": null,
1340"height": null,
1341"justify_content": null,
1342"justify_items": null,
1343"left": null,
1344"margin": null,
1345"max_height": null,
1346"max_width": null,
1347"min_height": null,
1348"min_width": null,
1349"object_fit": null,
1350"object_position": null,
1351"order": null,
1352"overflow": null,
1353"overflow_x": null,
1354"overflow_y": null,
1355"padding": null,
1356"right": null,
1357"top": null,
1358"visibility": null,
1359"width": null
1360}
1361},
1362"33b5df6b101a4b16bcd0fbb13c8980d9": {
1363"model_module": "@jupyter-widgets/base",
1364"model_module_version": "1.2.0",
1365"model_name": "LayoutModel",
1366"state": {
1367"_model_module": "@jupyter-widgets/base",
1368"_model_module_version": "1.2.0",
1369"_model_name": "LayoutModel",
1370"_view_count": null,
1371"_view_module": "@jupyter-widgets/base",
1372"_view_module_version": "1.2.0",
1373"_view_name": "LayoutView",
1374"align_content": null,
1375"align_items": null,
1376"align_self": null,
1377"border": null,
1378"bottom": null,
1379"display": null,
1380"flex": null,
1381"flex_flow": null,
1382"grid_area": null,
1383"grid_auto_columns": null,
1384"grid_auto_flow": null,
1385"grid_auto_rows": null,
1386"grid_column": null,
1387"grid_gap": null,
1388"grid_row": null,
1389"grid_template_areas": null,
1390"grid_template_columns": null,
1391"grid_template_rows": null,
1392"height": null,
1393"justify_content": null,
1394"justify_items": null,
1395"left": null,
1396"margin": null,
1397"max_height": null,
1398"max_width": null,
1399"min_height": null,
1400"min_width": null,
1401"object_fit": null,
1402"object_position": null,
1403"order": null,
1404"overflow": null,
1405"overflow_x": null,
1406"overflow_y": null,
1407"padding": null,
1408"right": null,
1409"top": null,
1410"visibility": null,
1411"width": null
1412}
1413},
1414"33c13d2da30c47b18c77a1cef006497d": {
1415"model_module": "@jupyter-widgets/controls",
1416"model_module_version": "1.5.0",
1417"model_name": "DescriptionStyleModel",
1418"state": {
1419"_model_module": "@jupyter-widgets/controls",
1420"_model_module_version": "1.5.0",
1421"_model_name": "DescriptionStyleModel",
1422"_view_count": null,
1423"_view_module": "@jupyter-widgets/base",
1424"_view_module_version": "1.2.0",
1425"_view_name": "StyleView",
1426"description_width": ""
1427}
1428},
1429"353d4c638c3b4ac2b6bd0411fd6b1dca": {
1430"model_module": "@jupyter-widgets/controls",
1431"model_module_version": "1.5.0",
1432"model_name": "HTMLModel",
1433"state": {
1434"_dom_classes": [],
1435"_model_module": "@jupyter-widgets/controls",
1436"_model_module_version": "1.5.0",
1437"_model_name": "HTMLModel",
1438"_view_count": null,
1439"_view_module": "@jupyter-widgets/controls",
1440"_view_module_version": "1.5.0",
1441"_view_name": "HTMLView",
1442"description": "",
1443"description_tooltip": null,
1444"layout": "IPY_MODEL_ad54965c74384ab283e7c638d254d763",
1445"placeholder": "",
1446"style": "IPY_MODEL_ef2447b1e70d499ebc080c4e3b46f35c",
1447"value": " 1/1 [00:00<00:00, 20.89it/s]"
1448}
1449},
1450"38e8dfef6cbf4f2b82471b74d3814177": {
1451"model_module": "@jupyter-widgets/controls",
1452"model_module_version": "1.5.0",
1453"model_name": "DescriptionStyleModel",
1454"state": {
1455"_model_module": "@jupyter-widgets/controls",
1456"_model_module_version": "1.5.0",
1457"_model_name": "DescriptionStyleModel",
1458"_view_count": null,
1459"_view_module": "@jupyter-widgets/base",
1460"_view_module_version": "1.2.0",
1461"_view_name": "StyleView",
1462"description_width": ""
1463}
1464},
1465"3904531861a44de996c9a48170d06f7e": {
1466"model_module": "@jupyter-widgets/controls",
1467"model_module_version": "1.5.0",
1468"model_name": "HBoxModel",
1469"state": {
1470"_dom_classes": [],
1471"_model_module": "@jupyter-widgets/controls",
1472"_model_module_version": "1.5.0",
1473"_model_name": "HBoxModel",
1474"_view_count": null,
1475"_view_module": "@jupyter-widgets/controls",
1476"_view_module_version": "1.5.0",
1477"_view_name": "HBoxView",
1478"box_style": "",
1479"children": [
1480"IPY_MODEL_0253c9956d434314bc7903c5b0123530",
1481"IPY_MODEL_39c38fff58154f8291a4635387230036",
1482"IPY_MODEL_c7d62339c034489b8dd9ade762892ff8"
1483],
1484"layout": "IPY_MODEL_dbb57469064a479085473de8e71ce4d0"
1485}
1486},
1487"39c38fff58154f8291a4635387230036": {
1488"model_module": "@jupyter-widgets/controls",
1489"model_module_version": "1.5.0",
1490"model_name": "FloatProgressModel",
1491"state": {
1492"_dom_classes": [],
1493"_model_module": "@jupyter-widgets/controls",
1494"_model_module_version": "1.5.0",
1495"_model_name": "FloatProgressModel",
1496"_view_count": null,
1497"_view_module": "@jupyter-widgets/controls",
1498"_view_module_version": "1.5.0",
1499"_view_name": "ProgressView",
1500"bar_style": "success",
1501"description": "",
1502"description_tooltip": null,
1503"layout": "IPY_MODEL_c050d37c2f4642b0925abd9e2eb06d99",
1504"max": 1,
1505"min": 0,
1506"orientation": "horizontal",
1507"style": "IPY_MODEL_a1022f00dda143778c1a1ebc80f7e878",
1508"value": 1
1509}
1510},
1511"3ee68111e3844c70a6ea72f295ef0392": {
1512"model_module": "@jupyter-widgets/controls",
1513"model_module_version": "1.5.0",
1514"model_name": "FloatProgressModel",
1515"state": {
1516"_dom_classes": [],
1517"_model_module": "@jupyter-widgets/controls",
1518"_model_module_version": "1.5.0",
1519"_model_name": "FloatProgressModel",
1520"_view_count": null,
1521"_view_module": "@jupyter-widgets/controls",
1522"_view_module_version": "1.5.0",
1523"_view_name": "ProgressView",
1524"bar_style": "success",
1525"description": "",
1526"description_tooltip": null,
1527"layout": "IPY_MODEL_93a607e6574f4a0fb3b9b1463a4aad4c",
1528"max": 1,
1529"min": 0,
1530"orientation": "horizontal",
1531"style": "IPY_MODEL_8c4d1d61155e4064bdf76599be6c8bbe",
1532"value": 1
1533}
1534},
1535"3ef8a6849b23425ba3074c6353c58f83": {
1536"model_module": "@jupyter-widgets/controls",
1537"model_module_version": "1.5.0",
1538"model_name": "HTMLModel",
1539"state": {
1540"_dom_classes": [],
1541"_model_module": "@jupyter-widgets/controls",
1542"_model_module_version": "1.5.0",
1543"_model_name": "HTMLModel",
1544"_view_count": null,
1545"_view_module": "@jupyter-widgets/controls",
1546"_view_module_version": "1.5.0",
1547"_view_name": "HTMLView",
1548"description": "",
1549"description_tooltip": null,
1550"layout": "IPY_MODEL_035f6e1399144c2a8cb45b8b5be92f32",
1551"placeholder": "",
1552"style": "IPY_MODEL_dbd48e8c1a9445e3b757609cf0b60fa3",
1553"value": " 1/1 [00:00<00:00, 24.14it/s]"
1554}
1555},
1556"3fa2749682e84da5b073250e391e9970": {
1557"model_module": "@jupyter-widgets/controls",
1558"model_module_version": "1.5.0",
1559"model_name": "HTMLModel",
1560"state": {
1561"_dom_classes": [],
1562"_model_module": "@jupyter-widgets/controls",
1563"_model_module_version": "1.5.0",
1564"_model_name": "HTMLModel",
1565"_view_count": null,
1566"_view_module": "@jupyter-widgets/controls",
1567"_view_module_version": "1.5.0",
1568"_view_name": "HTMLView",
1569"description": "",
1570"description_tooltip": null,
1571"layout": "IPY_MODEL_4447f0b788e944c184ca8b885a105d61",
1572"placeholder": "",
1573"style": "IPY_MODEL_e530c06891274d41b5d8a2b20e160ee4",
1574"value": " 1/1 [00:00<00:00, 23.17it/s]"
1575}
1576},
1577"3ff3b8f606f44adfa413314526d0f51b": {
1578"model_module": "@jupyter-widgets/controls",
1579"model_module_version": "1.5.0",
1580"model_name": "HBoxModel",
1581"state": {
1582"_dom_classes": [],
1583"_model_module": "@jupyter-widgets/controls",
1584"_model_module_version": "1.5.0",
1585"_model_name": "HBoxModel",
1586"_view_count": null,
1587"_view_module": "@jupyter-widgets/controls",
1588"_view_module_version": "1.5.0",
1589"_view_name": "HBoxView",
1590"box_style": "",
1591"children": [
1592"IPY_MODEL_4b436abd81db41ad98f24f3148bb1fcb",
1593"IPY_MODEL_a20d8628bdb5486a8ccab95660a986c3",
1594"IPY_MODEL_42c2a9dbc747478e9f4691d01f1246da"
1595],
1596"layout": "IPY_MODEL_616af910d6ba45039b3b455631fe545c"
1597}
1598},
1599"422ce6e179ca44439e0e1bb574daecc8": {
1600"model_module": "@jupyter-widgets/base",
1601"model_module_version": "1.2.0",
1602"model_name": "LayoutModel",
1603"state": {
1604"_model_module": "@jupyter-widgets/base",
1605"_model_module_version": "1.2.0",
1606"_model_name": "LayoutModel",
1607"_view_count": null,
1608"_view_module": "@jupyter-widgets/base",
1609"_view_module_version": "1.2.0",
1610"_view_name": "LayoutView",
1611"align_content": null,
1612"align_items": null,
1613"align_self": null,
1614"border": null,
1615"bottom": null,
1616"display": null,
1617"flex": null,
1618"flex_flow": null,
1619"grid_area": null,
1620"grid_auto_columns": null,
1621"grid_auto_flow": null,
1622"grid_auto_rows": null,
1623"grid_column": null,
1624"grid_gap": null,
1625"grid_row": null,
1626"grid_template_areas": null,
1627"grid_template_columns": null,
1628"grid_template_rows": null,
1629"height": null,
1630"justify_content": null,
1631"justify_items": null,
1632"left": null,
1633"margin": null,
1634"max_height": null,
1635"max_width": null,
1636"min_height": null,
1637"min_width": null,
1638"object_fit": null,
1639"object_position": null,
1640"order": null,
1641"overflow": null,
1642"overflow_x": null,
1643"overflow_y": null,
1644"padding": null,
1645"right": null,
1646"top": null,
1647"visibility": null,
1648"width": null
1649}
1650},
1651"429276694dba48d2a5958f4945ede4b7": {
1652"model_module": "@jupyter-widgets/base",
1653"model_module_version": "1.2.0",
1654"model_name": "LayoutModel",
1655"state": {
1656"_model_module": "@jupyter-widgets/base",
1657"_model_module_version": "1.2.0",
1658"_model_name": "LayoutModel",
1659"_view_count": null,
1660"_view_module": "@jupyter-widgets/base",
1661"_view_module_version": "1.2.0",
1662"_view_name": "LayoutView",
1663"align_content": null,
1664"align_items": null,
1665"align_self": null,
1666"border": null,
1667"bottom": null,
1668"display": null,
1669"flex": null,
1670"flex_flow": null,
1671"grid_area": null,
1672"grid_auto_columns": null,
1673"grid_auto_flow": null,
1674"grid_auto_rows": null,
1675"grid_column": null,
1676"grid_gap": null,
1677"grid_row": null,
1678"grid_template_areas": null,
1679"grid_template_columns": null,
1680"grid_template_rows": null,
1681"height": null,
1682"justify_content": null,
1683"justify_items": null,
1684"left": null,
1685"margin": null,
1686"max_height": null,
1687"max_width": null,
1688"min_height": null,
1689"min_width": null,
1690"object_fit": null,
1691"object_position": null,
1692"order": null,
1693"overflow": null,
1694"overflow_x": null,
1695"overflow_y": null,
1696"padding": null,
1697"right": null,
1698"top": null,
1699"visibility": null,
1700"width": null
1701}
1702},
1703"42c2a9dbc747478e9f4691d01f1246da": {
1704"model_module": "@jupyter-widgets/controls",
1705"model_module_version": "1.5.0",
1706"model_name": "HTMLModel",
1707"state": {
1708"_dom_classes": [],
1709"_model_module": "@jupyter-widgets/controls",
1710"_model_module_version": "1.5.0",
1711"_model_name": "HTMLModel",
1712"_view_count": null,
1713"_view_module": "@jupyter-widgets/controls",
1714"_view_module_version": "1.5.0",
1715"_view_name": "HTMLView",
1716"description": "",
1717"description_tooltip": null,
1718"layout": "IPY_MODEL_4afe18f598764e90807ac2f32fca4853",
1719"placeholder": "",
1720"style": "IPY_MODEL_63850c8393aa4c1aa8a5e195857ceca3",
1721"value": " 1/1 [00:00<00:00, 2.17 Batches/s]"
1722}
1723},
1724"4402e159d59a4518a894d7ded2025b21": {
1725"model_module": "@jupyter-widgets/controls",
1726"model_module_version": "1.5.0",
1727"model_name": "HTMLModel",
1728"state": {
1729"_dom_classes": [],
1730"_model_module": "@jupyter-widgets/controls",
1731"_model_module_version": "1.5.0",
1732"_model_name": "HTMLModel",
1733"_view_count": null,
1734"_view_module": "@jupyter-widgets/controls",
1735"_view_module_version": "1.5.0",
1736"_view_name": "HTMLView",
1737"description": "",
1738"description_tooltip": null,
1739"layout": "IPY_MODEL_c4539733aed34c7b940de5da7edfa82a",
1740"placeholder": "",
1741"style": "IPY_MODEL_2a0ebf357d0f4ed3b283b561e5ab5774",
1742"value": "Batches: 100%"
1743}
1744},
1745"4447f0b788e944c184ca8b885a105d61": {
1746"model_module": "@jupyter-widgets/base",
1747"model_module_version": "1.2.0",
1748"model_name": "LayoutModel",
1749"state": {
1750"_model_module": "@jupyter-widgets/base",
1751"_model_module_version": "1.2.0",
1752"_model_name": "LayoutModel",
1753"_view_count": null,
1754"_view_module": "@jupyter-widgets/base",
1755"_view_module_version": "1.2.0",
1756"_view_name": "LayoutView",
1757"align_content": null,
1758"align_items": null,
1759"align_self": null,
1760"border": null,
1761"bottom": null,
1762"display": null,
1763"flex": null,
1764"flex_flow": null,
1765"grid_area": null,
1766"grid_auto_columns": null,
1767"grid_auto_flow": null,
1768"grid_auto_rows": null,
1769"grid_column": null,
1770"grid_gap": null,
1771"grid_row": null,
1772"grid_template_areas": null,
1773"grid_template_columns": null,
1774"grid_template_rows": null,
1775"height": null,
1776"justify_content": null,
1777"justify_items": null,
1778"left": null,
1779"margin": null,
1780"max_height": null,
1781"max_width": null,
1782"min_height": null,
1783"min_width": null,
1784"object_fit": null,
1785"object_position": null,
1786"order": null,
1787"overflow": null,
1788"overflow_x": null,
1789"overflow_y": null,
1790"padding": null,
1791"right": null,
1792"top": null,
1793"visibility": null,
1794"width": null
1795}
1796},
1797"4afe18f598764e90807ac2f32fca4853": {
1798"model_module": "@jupyter-widgets/base",
1799"model_module_version": "1.2.0",
1800"model_name": "LayoutModel",
1801"state": {
1802"_model_module": "@jupyter-widgets/base",
1803"_model_module_version": "1.2.0",
1804"_model_name": "LayoutModel",
1805"_view_count": null,
1806"_view_module": "@jupyter-widgets/base",
1807"_view_module_version": "1.2.0",
1808"_view_name": "LayoutView",
1809"align_content": null,
1810"align_items": null,
1811"align_self": null,
1812"border": null,
1813"bottom": null,
1814"display": null,
1815"flex": null,
1816"flex_flow": null,
1817"grid_area": null,
1818"grid_auto_columns": null,
1819"grid_auto_flow": null,
1820"grid_auto_rows": null,
1821"grid_column": null,
1822"grid_gap": null,
1823"grid_row": null,
1824"grid_template_areas": null,
1825"grid_template_columns": null,
1826"grid_template_rows": null,
1827"height": null,
1828"justify_content": null,
1829"justify_items": null,
1830"left": null,
1831"margin": null,
1832"max_height": null,
1833"max_width": null,
1834"min_height": null,
1835"min_width": null,
1836"object_fit": null,
1837"object_position": null,
1838"order": null,
1839"overflow": null,
1840"overflow_x": null,
1841"overflow_y": null,
1842"padding": null,
1843"right": null,
1844"top": null,
1845"visibility": null,
1846"width": null
1847}
1848},
1849"4b436abd81db41ad98f24f3148bb1fcb": {
1850"model_module": "@jupyter-widgets/controls",
1851"model_module_version": "1.5.0",
1852"model_name": "HTMLModel",
1853"state": {
1854"_dom_classes": [],
1855"_model_module": "@jupyter-widgets/controls",
1856"_model_module_version": "1.5.0",
1857"_model_name": "HTMLModel",
1858"_view_count": null,
1859"_view_module": "@jupyter-widgets/controls",
1860"_view_module_version": "1.5.0",
1861"_view_name": "HTMLView",
1862"description": "",
1863"description_tooltip": null,
1864"layout": "IPY_MODEL_422ce6e179ca44439e0e1bb574daecc8",
1865"placeholder": "",
1866"style": "IPY_MODEL_5f05f9f76d5149e0a3c42e08dd85a82b",
1867"value": "Inferencing Samples: 100%"
1868}
1869},
1870"4c41e83814e44a578a6e62585cafd34d": {
1871"model_module": "@jupyter-widgets/controls",
1872"model_module_version": "1.5.0",
1873"model_name": "ProgressStyleModel",
1874"state": {
1875"_model_module": "@jupyter-widgets/controls",
1876"_model_module_version": "1.5.0",
1877"_model_name": "ProgressStyleModel",
1878"_view_count": null,
1879"_view_module": "@jupyter-widgets/base",
1880"_view_module_version": "1.2.0",
1881"_view_name": "StyleView",
1882"bar_color": null,
1883"description_width": ""
1884}
1885},
1886"4c514834225147eab4ba48b7ece93ecb": {
1887"model_module": "@jupyter-widgets/base",
1888"model_module_version": "1.2.0",
1889"model_name": "LayoutModel",
1890"state": {
1891"_model_module": "@jupyter-widgets/base",
1892"_model_module_version": "1.2.0",
1893"_model_name": "LayoutModel",
1894"_view_count": null,
1895"_view_module": "@jupyter-widgets/base",
1896"_view_module_version": "1.2.0",
1897"_view_name": "LayoutView",
1898"align_content": null,
1899"align_items": null,
1900"align_self": null,
1901"border": null,
1902"bottom": null,
1903"display": null,
1904"flex": null,
1905"flex_flow": null,
1906"grid_area": null,
1907"grid_auto_columns": null,
1908"grid_auto_flow": null,
1909"grid_auto_rows": null,
1910"grid_column": null,
1911"grid_gap": null,
1912"grid_row": null,
1913"grid_template_areas": null,
1914"grid_template_columns": null,
1915"grid_template_rows": null,
1916"height": null,
1917"justify_content": null,
1918"justify_items": null,
1919"left": null,
1920"margin": null,
1921"max_height": null,
1922"max_width": null,
1923"min_height": null,
1924"min_width": null,
1925"object_fit": null,
1926"object_position": null,
1927"order": null,
1928"overflow": null,
1929"overflow_x": null,
1930"overflow_y": null,
1931"padding": null,
1932"right": null,
1933"top": null,
1934"visibility": null,
1935"width": null
1936}
1937},
1938"55405182fbc24485934754b53eac871b": {
1939"model_module": "@jupyter-widgets/controls",
1940"model_module_version": "1.5.0",
1941"model_name": "ProgressStyleModel",
1942"state": {
1943"_model_module": "@jupyter-widgets/controls",
1944"_model_module_version": "1.5.0",
1945"_model_name": "ProgressStyleModel",
1946"_view_count": null,
1947"_view_module": "@jupyter-widgets/base",
1948"_view_module_version": "1.2.0",
1949"_view_name": "StyleView",
1950"bar_color": null,
1951"description_width": ""
1952}
1953},
1954"579ff5067d6b46c893612bd233906b34": {
1955"model_module": "@jupyter-widgets/controls",
1956"model_module_version": "1.5.0",
1957"model_name": "HBoxModel",
1958"state": {
1959"_dom_classes": [],
1960"_model_module": "@jupyter-widgets/controls",
1961"_model_module_version": "1.5.0",
1962"_model_name": "HBoxModel",
1963"_view_count": null,
1964"_view_module": "@jupyter-widgets/controls",
1965"_view_module_version": "1.5.0",
1966"_view_name": "HBoxView",
1967"box_style": "",
1968"children": [
1969"IPY_MODEL_9a4a31fda4e64a27ae2c884ad1e9d9c9",
1970"IPY_MODEL_3ee68111e3844c70a6ea72f295ef0392",
1971"IPY_MODEL_d5c66b0a453f4a03954fabfd85187e83"
1972],
1973"layout": "IPY_MODEL_650915e12d2644c38cf2f6ec72faba92"
1974}
1975},
1976"5b64bdd8ce404f8290511d9bf9c7f29f": {
1977"model_module": "@jupyter-widgets/controls",
1978"model_module_version": "1.5.0",
1979"model_name": "DescriptionStyleModel",
1980"state": {
1981"_model_module": "@jupyter-widgets/controls",
1982"_model_module_version": "1.5.0",
1983"_model_name": "DescriptionStyleModel",
1984"_view_count": null,
1985"_view_module": "@jupyter-widgets/base",
1986"_view_module_version": "1.2.0",
1987"_view_name": "StyleView",
1988"description_width": ""
1989}
1990},
1991"5c01678b9ac64366b728da1cecd4e1c0": {
1992"model_module": "@jupyter-widgets/base",
1993"model_module_version": "1.2.0",
1994"model_name": "LayoutModel",
1995"state": {
1996"_model_module": "@jupyter-widgets/base",
1997"_model_module_version": "1.2.0",
1998"_model_name": "LayoutModel",
1999"_view_count": null,
2000"_view_module": "@jupyter-widgets/base",
2001"_view_module_version": "1.2.0",
2002"_view_name": "LayoutView",
2003"align_content": null,
2004"align_items": null,
2005"align_self": null,
2006"border": null,
2007"bottom": null,
2008"display": null,
2009"flex": null,
2010"flex_flow": null,
2011"grid_area": null,
2012"grid_auto_columns": null,
2013"grid_auto_flow": null,
2014"grid_auto_rows": null,
2015"grid_column": null,
2016"grid_gap": null,
2017"grid_row": null,
2018"grid_template_areas": null,
2019"grid_template_columns": null,
2020"grid_template_rows": null,
2021"height": null,
2022"justify_content": null,
2023"justify_items": null,
2024"left": null,
2025"margin": null,
2026"max_height": null,
2027"max_width": null,
2028"min_height": null,
2029"min_width": null,
2030"object_fit": null,
2031"object_position": null,
2032"order": null,
2033"overflow": null,
2034"overflow_x": null,
2035"overflow_y": null,
2036"padding": null,
2037"right": null,
2038"top": null,
2039"visibility": null,
2040"width": null
2041}
2042},
2043"5f05f9f76d5149e0a3c42e08dd85a82b": {
2044"model_module": "@jupyter-widgets/controls",
2045"model_module_version": "1.5.0",
2046"model_name": "DescriptionStyleModel",
2047"state": {
2048"_model_module": "@jupyter-widgets/controls",
2049"_model_module_version": "1.5.0",
2050"_model_name": "DescriptionStyleModel",
2051"_view_count": null,
2052"_view_module": "@jupyter-widgets/base",
2053"_view_module_version": "1.2.0",
2054"_view_name": "StyleView",
2055"description_width": ""
2056}
2057},
2058"5f537475318e43e4b3b7e54b43a978b5": {
2059"model_module": "@jupyter-widgets/controls",
2060"model_module_version": "1.5.0",
2061"model_name": "DescriptionStyleModel",
2062"state": {
2063"_model_module": "@jupyter-widgets/controls",
2064"_model_module_version": "1.5.0",
2065"_model_name": "DescriptionStyleModel",
2066"_view_count": null,
2067"_view_module": "@jupyter-widgets/base",
2068"_view_module_version": "1.2.0",
2069"_view_name": "StyleView",
2070"description_width": ""
2071}
2072},
2073"5f8dea9534fd47e9a1efddecc4e6da3d": {
2074"model_module": "@jupyter-widgets/base",
2075"model_module_version": "1.2.0",
2076"model_name": "LayoutModel",
2077"state": {
2078"_model_module": "@jupyter-widgets/base",
2079"_model_module_version": "1.2.0",
2080"_model_name": "LayoutModel",
2081"_view_count": null,
2082"_view_module": "@jupyter-widgets/base",
2083"_view_module_version": "1.2.0",
2084"_view_name": "LayoutView",
2085"align_content": null,
2086"align_items": null,
2087"align_self": null,
2088"border": null,
2089"bottom": null,
2090"display": null,
2091"flex": null,
2092"flex_flow": null,
2093"grid_area": null,
2094"grid_auto_columns": null,
2095"grid_auto_flow": null,
2096"grid_auto_rows": null,
2097"grid_column": null,
2098"grid_gap": null,
2099"grid_row": null,
2100"grid_template_areas": null,
2101"grid_template_columns": null,
2102"grid_template_rows": null,
2103"height": null,
2104"justify_content": null,
2105"justify_items": null,
2106"left": null,
2107"margin": null,
2108"max_height": null,
2109"max_width": null,
2110"min_height": null,
2111"min_width": null,
2112"object_fit": null,
2113"object_position": null,
2114"order": null,
2115"overflow": null,
2116"overflow_x": null,
2117"overflow_y": null,
2118"padding": null,
2119"right": null,
2120"top": null,
2121"visibility": null,
2122"width": null
2123}
2124},
2125"616af910d6ba45039b3b455631fe545c": {
2126"model_module": "@jupyter-widgets/base",
2127"model_module_version": "1.2.0",
2128"model_name": "LayoutModel",
2129"state": {
2130"_model_module": "@jupyter-widgets/base",
2131"_model_module_version": "1.2.0",
2132"_model_name": "LayoutModel",
2133"_view_count": null,
2134"_view_module": "@jupyter-widgets/base",
2135"_view_module_version": "1.2.0",
2136"_view_name": "LayoutView",
2137"align_content": null,
2138"align_items": null,
2139"align_self": null,
2140"border": null,
2141"bottom": null,
2142"display": null,
2143"flex": null,
2144"flex_flow": null,
2145"grid_area": null,
2146"grid_auto_columns": null,
2147"grid_auto_flow": null,
2148"grid_auto_rows": null,
2149"grid_column": null,
2150"grid_gap": null,
2151"grid_row": null,
2152"grid_template_areas": null,
2153"grid_template_columns": null,
2154"grid_template_rows": null,
2155"height": null,
2156"justify_content": null,
2157"justify_items": null,
2158"left": null,
2159"margin": null,
2160"max_height": null,
2161"max_width": null,
2162"min_height": null,
2163"min_width": null,
2164"object_fit": null,
2165"object_position": null,
2166"order": null,
2167"overflow": null,
2168"overflow_x": null,
2169"overflow_y": null,
2170"padding": null,
2171"right": null,
2172"top": null,
2173"visibility": null,
2174"width": null
2175}
2176},
2177"633687b1bf3e49b2bbe5c8fcdf21b187": {
2178"model_module": "@jupyter-widgets/base",
2179"model_module_version": "1.2.0",
2180"model_name": "LayoutModel",
2181"state": {
2182"_model_module": "@jupyter-widgets/base",
2183"_model_module_version": "1.2.0",
2184"_model_name": "LayoutModel",
2185"_view_count": null,
2186"_view_module": "@jupyter-widgets/base",
2187"_view_module_version": "1.2.0",
2188"_view_name": "LayoutView",
2189"align_content": null,
2190"align_items": null,
2191"align_self": null,
2192"border": null,
2193"bottom": null,
2194"display": null,
2195"flex": null,
2196"flex_flow": null,
2197"grid_area": null,
2198"grid_auto_columns": null,
2199"grid_auto_flow": null,
2200"grid_auto_rows": null,
2201"grid_column": null,
2202"grid_gap": null,
2203"grid_row": null,
2204"grid_template_areas": null,
2205"grid_template_columns": null,
2206"grid_template_rows": null,
2207"height": null,
2208"justify_content": null,
2209"justify_items": null,
2210"left": null,
2211"margin": null,
2212"max_height": null,
2213"max_width": null,
2214"min_height": null,
2215"min_width": null,
2216"object_fit": null,
2217"object_position": null,
2218"order": null,
2219"overflow": null,
2220"overflow_x": null,
2221"overflow_y": null,
2222"padding": null,
2223"right": null,
2224"top": null,
2225"visibility": null,
2226"width": null
2227}
2228},
2229"63850c8393aa4c1aa8a5e195857ceca3": {
2230"model_module": "@jupyter-widgets/controls",
2231"model_module_version": "1.5.0",
2232"model_name": "DescriptionStyleModel",
2233"state": {
2234"_model_module": "@jupyter-widgets/controls",
2235"_model_module_version": "1.5.0",
2236"_model_name": "DescriptionStyleModel",
2237"_view_count": null,
2238"_view_module": "@jupyter-widgets/base",
2239"_view_module_version": "1.2.0",
2240"_view_name": "StyleView",
2241"description_width": ""
2242}
2243},
2244"650915e12d2644c38cf2f6ec72faba92": {
2245"model_module": "@jupyter-widgets/base",
2246"model_module_version": "1.2.0",
2247"model_name": "LayoutModel",
2248"state": {
2249"_model_module": "@jupyter-widgets/base",
2250"_model_module_version": "1.2.0",
2251"_model_name": "LayoutModel",
2252"_view_count": null,
2253"_view_module": "@jupyter-widgets/base",
2254"_view_module_version": "1.2.0",
2255"_view_name": "LayoutView",
2256"align_content": null,
2257"align_items": null,
2258"align_self": null,
2259"border": null,
2260"bottom": null,
2261"display": null,
2262"flex": null,
2263"flex_flow": null,
2264"grid_area": null,
2265"grid_auto_columns": null,
2266"grid_auto_flow": null,
2267"grid_auto_rows": null,
2268"grid_column": null,
2269"grid_gap": null,
2270"grid_row": null,
2271"grid_template_areas": null,
2272"grid_template_columns": null,
2273"grid_template_rows": null,
2274"height": null,
2275"justify_content": null,
2276"justify_items": null,
2277"left": null,
2278"margin": null,
2279"max_height": null,
2280"max_width": null,
2281"min_height": null,
2282"min_width": null,
2283"object_fit": null,
2284"object_position": null,
2285"order": null,
2286"overflow": null,
2287"overflow_x": null,
2288"overflow_y": null,
2289"padding": null,
2290"right": null,
2291"top": null,
2292"visibility": null,
2293"width": null
2294}
2295},
2296"68b55859ea7b420a9bd917b85d122864": {
2297"model_module": "@jupyter-widgets/controls",
2298"model_module_version": "1.5.0",
2299"model_name": "HTMLModel",
2300"state": {
2301"_dom_classes": [],
2302"_model_module": "@jupyter-widgets/controls",
2303"_model_module_version": "1.5.0",
2304"_model_name": "HTMLModel",
2305"_view_count": null,
2306"_view_module": "@jupyter-widgets/controls",
2307"_view_module_version": "1.5.0",
2308"_view_name": "HTMLView",
2309"description": "",
2310"description_tooltip": null,
2311"layout": "IPY_MODEL_9e52e775dc5640be920eebc454aaa67c",
2312"placeholder": "",
2313"style": "IPY_MODEL_8f3b6fb4b42f4382a6de2017fb79f5b8",
2314"value": "Batches: 100%"
2315}
2316},
2317"6baf2644608242a6a431cd3939201d51": {
2318"model_module": "@jupyter-widgets/base",
2319"model_module_version": "1.2.0",
2320"model_name": "LayoutModel",
2321"state": {
2322"_model_module": "@jupyter-widgets/base",
2323"_model_module_version": "1.2.0",
2324"_model_name": "LayoutModel",
2325"_view_count": null,
2326"_view_module": "@jupyter-widgets/base",
2327"_view_module_version": "1.2.0",
2328"_view_name": "LayoutView",
2329"align_content": null,
2330"align_items": null,
2331"align_self": null,
2332"border": null,
2333"bottom": null,
2334"display": null,
2335"flex": null,
2336"flex_flow": null,
2337"grid_area": null,
2338"grid_auto_columns": null,
2339"grid_auto_flow": null,
2340"grid_auto_rows": null,
2341"grid_column": null,
2342"grid_gap": null,
2343"grid_row": null,
2344"grid_template_areas": null,
2345"grid_template_columns": null,
2346"grid_template_rows": null,
2347"height": null,
2348"justify_content": null,
2349"justify_items": null,
2350"left": null,
2351"margin": null,
2352"max_height": null,
2353"max_width": null,
2354"min_height": null,
2355"min_width": null,
2356"object_fit": null,
2357"object_position": null,
2358"order": null,
2359"overflow": null,
2360"overflow_x": null,
2361"overflow_y": null,
2362"padding": null,
2363"right": null,
2364"top": null,
2365"visibility": null,
2366"width": null
2367}
2368},
2369"6dcbaa9a020048119de893c5dd3fe5b3": {
2370"model_module": "@jupyter-widgets/controls",
2371"model_module_version": "1.5.0",
2372"model_name": "HTMLModel",
2373"state": {
2374"_dom_classes": [],
2375"_model_module": "@jupyter-widgets/controls",
2376"_model_module_version": "1.5.0",
2377"_model_name": "HTMLModel",
2378"_view_count": null,
2379"_view_module": "@jupyter-widgets/controls",
2380"_view_module_version": "1.5.0",
2381"_view_name": "HTMLView",
2382"description": "",
2383"description_tooltip": null,
2384"layout": "IPY_MODEL_5f8dea9534fd47e9a1efddecc4e6da3d",
2385"placeholder": "",
2386"style": "IPY_MODEL_33c13d2da30c47b18c77a1cef006497d",
2387"value": "Batches: 100%"
2388}
2389},
2390"6ee5d390d87b48888029b917805ac640": {
2391"model_module": "@jupyter-widgets/controls",
2392"model_module_version": "1.5.0",
2393"model_name": "DescriptionStyleModel",
2394"state": {
2395"_model_module": "@jupyter-widgets/controls",
2396"_model_module_version": "1.5.0",
2397"_model_name": "DescriptionStyleModel",
2398"_view_count": null,
2399"_view_module": "@jupyter-widgets/base",
2400"_view_module_version": "1.2.0",
2401"_view_name": "StyleView",
2402"description_width": ""
2403}
2404},
2405"7091ed26beba439690f78dfa715542c5": {
2406"model_module": "@jupyter-widgets/controls",
2407"model_module_version": "1.5.0",
2408"model_name": "FloatProgressModel",
2409"state": {
2410"_dom_classes": [],
2411"_model_module": "@jupyter-widgets/controls",
2412"_model_module_version": "1.5.0",
2413"_model_name": "FloatProgressModel",
2414"_view_count": null,
2415"_view_module": "@jupyter-widgets/controls",
2416"_view_module_version": "1.5.0",
2417"_view_name": "ProgressView",
2418"bar_style": "success",
2419"description": "",
2420"description_tooltip": null,
2421"layout": "IPY_MODEL_c72dbeb5557b4ac7bc46c957bab2485f",
2422"max": 1,
2423"min": 0,
2424"orientation": "horizontal",
2425"style": "IPY_MODEL_d8f756ab4c9245709c40a48df7842c07",
2426"value": 1
2427}
2428},
2429"7165f68ae5fd4b57a3f30942519f2d29": {
2430"model_module": "@jupyter-widgets/base",
2431"model_module_version": "1.2.0",
2432"model_name": "LayoutModel",
2433"state": {
2434"_model_module": "@jupyter-widgets/base",
2435"_model_module_version": "1.2.0",
2436"_model_name": "LayoutModel",
2437"_view_count": null,
2438"_view_module": "@jupyter-widgets/base",
2439"_view_module_version": "1.2.0",
2440"_view_name": "LayoutView",
2441"align_content": null,
2442"align_items": null,
2443"align_self": null,
2444"border": null,
2445"bottom": null,
2446"display": null,
2447"flex": null,
2448"flex_flow": null,
2449"grid_area": null,
2450"grid_auto_columns": null,
2451"grid_auto_flow": null,
2452"grid_auto_rows": null,
2453"grid_column": null,
2454"grid_gap": null,
2455"grid_row": null,
2456"grid_template_areas": null,
2457"grid_template_columns": null,
2458"grid_template_rows": null,
2459"height": null,
2460"justify_content": null,
2461"justify_items": null,
2462"left": null,
2463"margin": null,
2464"max_height": null,
2465"max_width": null,
2466"min_height": null,
2467"min_width": null,
2468"object_fit": null,
2469"object_position": null,
2470"order": null,
2471"overflow": null,
2472"overflow_x": null,
2473"overflow_y": null,
2474"padding": null,
2475"right": null,
2476"top": null,
2477"visibility": null,
2478"width": null
2479}
2480},
2481"729a5070b09f4b2aa511d58e2b5833d5": {
2482"model_module": "@jupyter-widgets/controls",
2483"model_module_version": "1.5.0",
2484"model_name": "HTMLModel",
2485"state": {
2486"_dom_classes": [],
2487"_model_module": "@jupyter-widgets/controls",
2488"_model_module_version": "1.5.0",
2489"_model_name": "HTMLModel",
2490"_view_count": null,
2491"_view_module": "@jupyter-widgets/controls",
2492"_view_module_version": "1.5.0",
2493"_view_name": "HTMLView",
2494"description": "",
2495"description_tooltip": null,
2496"layout": "IPY_MODEL_429276694dba48d2a5958f4945ede4b7",
2497"placeholder": "",
2498"style": "IPY_MODEL_95b8b7dcd2ff4152b8a684e5cb878a91",
2499"value": " 1/1 [00:00<00:00, 3.59 Batches/s]"
2500}
2501},
2502"77007e83bee849deb05fbfd2b9321310": {
2503"model_module": "@jupyter-widgets/controls",
2504"model_module_version": "1.5.0",
2505"model_name": "DescriptionStyleModel",
2506"state": {
2507"_model_module": "@jupyter-widgets/controls",
2508"_model_module_version": "1.5.0",
2509"_model_name": "DescriptionStyleModel",
2510"_view_count": null,
2511"_view_module": "@jupyter-widgets/base",
2512"_view_module_version": "1.2.0",
2513"_view_name": "StyleView",
2514"description_width": ""
2515}
2516},
2517"7ee82a73e82c4ad29a15bf336095fa49": {
2518"model_module": "@jupyter-widgets/controls",
2519"model_module_version": "1.5.0",
2520"model_name": "HTMLModel",
2521"state": {
2522"_dom_classes": [],
2523"_model_module": "@jupyter-widgets/controls",
2524"_model_module_version": "1.5.0",
2525"_model_name": "HTMLModel",
2526"_view_count": null,
2527"_view_module": "@jupyter-widgets/controls",
2528"_view_module_version": "1.5.0",
2529"_view_name": "HTMLView",
2530"description": "",
2531"description_tooltip": null,
2532"layout": "IPY_MODEL_812a04255c8648158e4f82665ff2efce",
2533"placeholder": "",
2534"style": "IPY_MODEL_f54f6e8998f54d5c8d367d244158c261",
2535"value": "Batches: 100%"
2536}
2537},
2538"7f57abc22d3a4231a6b02eb025b35f49": {
2539"model_module": "@jupyter-widgets/controls",
2540"model_module_version": "1.5.0",
2541"model_name": "HBoxModel",
2542"state": {
2543"_dom_classes": [],
2544"_model_module": "@jupyter-widgets/controls",
2545"_model_module_version": "1.5.0",
2546"_model_name": "HBoxModel",
2547"_view_count": null,
2548"_view_module": "@jupyter-widgets/controls",
2549"_view_module_version": "1.5.0",
2550"_view_name": "HBoxView",
2551"box_style": "",
2552"children": [
2553"IPY_MODEL_a9e34231df9548e584c81ea89b814ee6",
2554"IPY_MODEL_9989d5d79a3a41fa9bc0431465997611",
2555"IPY_MODEL_f73977385dce49bead6c2012f2e42f71"
2556],
2557"layout": "IPY_MODEL_cc2586d0b77349b2b68a0b05e410f95f"
2558}
2559},
2560"7f69e47ac44c401f9b26f8c88de4c481": {
2561"model_module": "@jupyter-widgets/controls",
2562"model_module_version": "1.5.0",
2563"model_name": "FloatProgressModel",
2564"state": {
2565"_dom_classes": [],
2566"_model_module": "@jupyter-widgets/controls",
2567"_model_module_version": "1.5.0",
2568"_model_name": "FloatProgressModel",
2569"_view_count": null,
2570"_view_module": "@jupyter-widgets/controls",
2571"_view_module_version": "1.5.0",
2572"_view_name": "ProgressView",
2573"bar_style": "success",
2574"description": "",
2575"description_tooltip": null,
2576"layout": "IPY_MODEL_be6dd7e2f43748d490deda9bab833ce4",
2577"max": 1,
2578"min": 0,
2579"orientation": "horizontal",
2580"style": "IPY_MODEL_8cdc612b706e4d0b8a2528d3176b575a",
2581"value": 1
2582}
2583},
2584"7fd49ced837540d2b3e560a6dd507ac3": {
2585"model_module": "@jupyter-widgets/controls",
2586"model_module_version": "1.5.0",
2587"model_name": "HTMLModel",
2588"state": {
2589"_dom_classes": [],
2590"_model_module": "@jupyter-widgets/controls",
2591"_model_module_version": "1.5.0",
2592"_model_name": "HTMLModel",
2593"_view_count": null,
2594"_view_module": "@jupyter-widgets/controls",
2595"_view_module_version": "1.5.0",
2596"_view_name": "HTMLView",
2597"description": "",
2598"description_tooltip": null,
2599"layout": "IPY_MODEL_c0cd6e5f853b4ee0858d3b4c53a102ee",
2600"placeholder": "",
2601"style": "IPY_MODEL_77007e83bee849deb05fbfd2b9321310",
2602"value": "Inferencing Samples: 100%"
2603}
2604},
2605"812a04255c8648158e4f82665ff2efce": {
2606"model_module": "@jupyter-widgets/base",
2607"model_module_version": "1.2.0",
2608"model_name": "LayoutModel",
2609"state": {
2610"_model_module": "@jupyter-widgets/base",
2611"_model_module_version": "1.2.0",
2612"_model_name": "LayoutModel",
2613"_view_count": null,
2614"_view_module": "@jupyter-widgets/base",
2615"_view_module_version": "1.2.0",
2616"_view_name": "LayoutView",
2617"align_content": null,
2618"align_items": null,
2619"align_self": null,
2620"border": null,
2621"bottom": null,
2622"display": null,
2623"flex": null,
2624"flex_flow": null,
2625"grid_area": null,
2626"grid_auto_columns": null,
2627"grid_auto_flow": null,
2628"grid_auto_rows": null,
2629"grid_column": null,
2630"grid_gap": null,
2631"grid_row": null,
2632"grid_template_areas": null,
2633"grid_template_columns": null,
2634"grid_template_rows": null,
2635"height": null,
2636"justify_content": null,
2637"justify_items": null,
2638"left": null,
2639"margin": null,
2640"max_height": null,
2641"max_width": null,
2642"min_height": null,
2643"min_width": null,
2644"object_fit": null,
2645"object_position": null,
2646"order": null,
2647"overflow": null,
2648"overflow_x": null,
2649"overflow_y": null,
2650"padding": null,
2651"right": null,
2652"top": null,
2653"visibility": null,
2654"width": null
2655}
2656},
2657"842019f6aed44d41976b11a0352d4596": {
2658"model_module": "@jupyter-widgets/controls",
2659"model_module_version": "1.5.0",
2660"model_name": "FloatProgressModel",
2661"state": {
2662"_dom_classes": [],
2663"_model_module": "@jupyter-widgets/controls",
2664"_model_module_version": "1.5.0",
2665"_model_name": "FloatProgressModel",
2666"_view_count": null,
2667"_view_module": "@jupyter-widgets/controls",
2668"_view_module_version": "1.5.0",
2669"_view_name": "ProgressView",
2670"bar_style": "success",
2671"description": "",
2672"description_tooltip": null,
2673"layout": "IPY_MODEL_d3d1971a3e3748c9ac7483faafbee1a0",
2674"max": 1,
2675"min": 0,
2676"orientation": "horizontal",
2677"style": "IPY_MODEL_25046a95109d4d25a2dd7bb70201c66d",
2678"value": 1
2679}
2680},
2681"85133ad2451b4f1db002b6b49a01f2d2": {
2682"model_module": "@jupyter-widgets/controls",
2683"model_module_version": "1.5.0",
2684"model_name": "HBoxModel",
2685"state": {
2686"_dom_classes": [],
2687"_model_module": "@jupyter-widgets/controls",
2688"_model_module_version": "1.5.0",
2689"_model_name": "HBoxModel",
2690"_view_count": null,
2691"_view_module": "@jupyter-widgets/controls",
2692"_view_module_version": "1.5.0",
2693"_view_name": "HBoxView",
2694"box_style": "",
2695"children": [
2696"IPY_MODEL_6dcbaa9a020048119de893c5dd3fe5b3",
2697"IPY_MODEL_9d4376e02eb7424ba13297548867ac44",
2698"IPY_MODEL_353d4c638c3b4ac2b6bd0411fd6b1dca"
2699],
2700"layout": "IPY_MODEL_054fa49e5ff8468eb24c38666a9783aa"
2701}
2702},
2703"8780553fdded4f0b906e0ce670a1657e": {
2704"model_module": "@jupyter-widgets/base",
2705"model_module_version": "1.2.0",
2706"model_name": "LayoutModel",
2707"state": {
2708"_model_module": "@jupyter-widgets/base",
2709"_model_module_version": "1.2.0",
2710"_model_name": "LayoutModel",
2711"_view_count": null,
2712"_view_module": "@jupyter-widgets/base",
2713"_view_module_version": "1.2.0",
2714"_view_name": "LayoutView",
2715"align_content": null,
2716"align_items": null,
2717"align_self": null,
2718"border": null,
2719"bottom": null,
2720"display": null,
2721"flex": null,
2722"flex_flow": null,
2723"grid_area": null,
2724"grid_auto_columns": null,
2725"grid_auto_flow": null,
2726"grid_auto_rows": null,
2727"grid_column": null,
2728"grid_gap": null,
2729"grid_row": null,
2730"grid_template_areas": null,
2731"grid_template_columns": null,
2732"grid_template_rows": null,
2733"height": null,
2734"justify_content": null,
2735"justify_items": null,
2736"left": null,
2737"margin": null,
2738"max_height": null,
2739"max_width": null,
2740"min_height": null,
2741"min_width": null,
2742"object_fit": null,
2743"object_position": null,
2744"order": null,
2745"overflow": null,
2746"overflow_x": null,
2747"overflow_y": null,
2748"padding": null,
2749"right": null,
2750"top": null,
2751"visibility": null,
2752"width": null
2753}
2754},
2755"897b099603c240f096b40b8da22f8aaa": {
2756"model_module": "@jupyter-widgets/base",
2757"model_module_version": "1.2.0",
2758"model_name": "LayoutModel",
2759"state": {
2760"_model_module": "@jupyter-widgets/base",
2761"_model_module_version": "1.2.0",
2762"_model_name": "LayoutModel",
2763"_view_count": null,
2764"_view_module": "@jupyter-widgets/base",
2765"_view_module_version": "1.2.0",
2766"_view_name": "LayoutView",
2767"align_content": null,
2768"align_items": null,
2769"align_self": null,
2770"border": null,
2771"bottom": null,
2772"display": null,
2773"flex": null,
2774"flex_flow": null,
2775"grid_area": null,
2776"grid_auto_columns": null,
2777"grid_auto_flow": null,
2778"grid_auto_rows": null,
2779"grid_column": null,
2780"grid_gap": null,
2781"grid_row": null,
2782"grid_template_areas": null,
2783"grid_template_columns": null,
2784"grid_template_rows": null,
2785"height": null,
2786"justify_content": null,
2787"justify_items": null,
2788"left": null,
2789"margin": null,
2790"max_height": null,
2791"max_width": null,
2792"min_height": null,
2793"min_width": null,
2794"object_fit": null,
2795"object_position": null,
2796"order": null,
2797"overflow": null,
2798"overflow_x": null,
2799"overflow_y": null,
2800"padding": null,
2801"right": null,
2802"top": null,
2803"visibility": null,
2804"width": null
2805}
2806},
2807"8c4d1d61155e4064bdf76599be6c8bbe": {
2808"model_module": "@jupyter-widgets/controls",
2809"model_module_version": "1.5.0",
2810"model_name": "ProgressStyleModel",
2811"state": {
2812"_model_module": "@jupyter-widgets/controls",
2813"_model_module_version": "1.5.0",
2814"_model_name": "ProgressStyleModel",
2815"_view_count": null,
2816"_view_module": "@jupyter-widgets/base",
2817"_view_module_version": "1.2.0",
2818"_view_name": "StyleView",
2819"bar_color": null,
2820"description_width": ""
2821}
2822},
2823"8cdc612b706e4d0b8a2528d3176b575a": {
2824"model_module": "@jupyter-widgets/controls",
2825"model_module_version": "1.5.0",
2826"model_name": "ProgressStyleModel",
2827"state": {
2828"_model_module": "@jupyter-widgets/controls",
2829"_model_module_version": "1.5.0",
2830"_model_name": "ProgressStyleModel",
2831"_view_count": null,
2832"_view_module": "@jupyter-widgets/base",
2833"_view_module_version": "1.2.0",
2834"_view_name": "StyleView",
2835"bar_color": null,
2836"description_width": ""
2837}
2838},
2839"8e01cbb665f442d494afc844eb4c1eac": {
2840"model_module": "@jupyter-widgets/controls",
2841"model_module_version": "1.5.0",
2842"model_name": "HBoxModel",
2843"state": {
2844"_dom_classes": [],
2845"_model_module": "@jupyter-widgets/controls",
2846"_model_module_version": "1.5.0",
2847"_model_name": "HBoxModel",
2848"_view_count": null,
2849"_view_module": "@jupyter-widgets/controls",
2850"_view_module_version": "1.5.0",
2851"_view_name": "HBoxView",
2852"box_style": "",
2853"children": [
2854"IPY_MODEL_68b55859ea7b420a9bd917b85d122864",
2855"IPY_MODEL_c88f10e17ab94da3852ae0f8ac7ff7db",
2856"IPY_MODEL_3ef8a6849b23425ba3074c6353c58f83"
2857],
2858"layout": "IPY_MODEL_c7673d0c64df414981cfc7246a0e01e6"
2859}
2860},
2861"8f3b6fb4b42f4382a6de2017fb79f5b8": {
2862"model_module": "@jupyter-widgets/controls",
2863"model_module_version": "1.5.0",
2864"model_name": "DescriptionStyleModel",
2865"state": {
2866"_model_module": "@jupyter-widgets/controls",
2867"_model_module_version": "1.5.0",
2868"_model_name": "DescriptionStyleModel",
2869"_view_count": null,
2870"_view_module": "@jupyter-widgets/base",
2871"_view_module_version": "1.2.0",
2872"_view_name": "StyleView",
2873"description_width": ""
2874}
2875},
2876"8fb5aba463ac40a18063b4fc7cd3d879": {
2877"model_module": "@jupyter-widgets/base",
2878"model_module_version": "1.2.0",
2879"model_name": "LayoutModel",
2880"state": {
2881"_model_module": "@jupyter-widgets/base",
2882"_model_module_version": "1.2.0",
2883"_model_name": "LayoutModel",
2884"_view_count": null,
2885"_view_module": "@jupyter-widgets/base",
2886"_view_module_version": "1.2.0",
2887"_view_name": "LayoutView",
2888"align_content": null,
2889"align_items": null,
2890"align_self": null,
2891"border": null,
2892"bottom": null,
2893"display": null,
2894"flex": null,
2895"flex_flow": null,
2896"grid_area": null,
2897"grid_auto_columns": null,
2898"grid_auto_flow": null,
2899"grid_auto_rows": null,
2900"grid_column": null,
2901"grid_gap": null,
2902"grid_row": null,
2903"grid_template_areas": null,
2904"grid_template_columns": null,
2905"grid_template_rows": null,
2906"height": null,
2907"justify_content": null,
2908"justify_items": null,
2909"left": null,
2910"margin": null,
2911"max_height": null,
2912"max_width": null,
2913"min_height": null,
2914"min_width": null,
2915"object_fit": null,
2916"object_position": null,
2917"order": null,
2918"overflow": null,
2919"overflow_x": null,
2920"overflow_y": null,
2921"padding": null,
2922"right": null,
2923"top": null,
2924"visibility": null,
2925"width": null
2926}
2927},
2928"9258bf70f05f4f3d96621a5c5a2a8a75": {
2929"model_module": "@jupyter-widgets/base",
2930"model_module_version": "1.2.0",
2931"model_name": "LayoutModel",
2932"state": {
2933"_model_module": "@jupyter-widgets/base",
2934"_model_module_version": "1.2.0",
2935"_model_name": "LayoutModel",
2936"_view_count": null,
2937"_view_module": "@jupyter-widgets/base",
2938"_view_module_version": "1.2.0",
2939"_view_name": "LayoutView",
2940"align_content": null,
2941"align_items": null,
2942"align_self": null,
2943"border": null,
2944"bottom": null,
2945"display": null,
2946"flex": null,
2947"flex_flow": null,
2948"grid_area": null,
2949"grid_auto_columns": null,
2950"grid_auto_flow": null,
2951"grid_auto_rows": null,
2952"grid_column": null,
2953"grid_gap": null,
2954"grid_row": null,
2955"grid_template_areas": null,
2956"grid_template_columns": null,
2957"grid_template_rows": null,
2958"height": null,
2959"justify_content": null,
2960"justify_items": null,
2961"left": null,
2962"margin": null,
2963"max_height": null,
2964"max_width": null,
2965"min_height": null,
2966"min_width": null,
2967"object_fit": null,
2968"object_position": null,
2969"order": null,
2970"overflow": null,
2971"overflow_x": null,
2972"overflow_y": null,
2973"padding": null,
2974"right": null,
2975"top": null,
2976"visibility": null,
2977"width": null
2978}
2979},
2980"936281119c65404f8af72d03b48a23a4": {
2981"model_module": "@jupyter-widgets/controls",
2982"model_module_version": "1.5.0",
2983"model_name": "HTMLModel",
2984"state": {
2985"_dom_classes": [],
2986"_model_module": "@jupyter-widgets/controls",
2987"_model_module_version": "1.5.0",
2988"_model_name": "HTMLModel",
2989"_view_count": null,
2990"_view_module": "@jupyter-widgets/controls",
2991"_view_module_version": "1.5.0",
2992"_view_name": "HTMLView",
2993"description": "",
2994"description_tooltip": null,
2995"layout": "IPY_MODEL_5c01678b9ac64366b728da1cecd4e1c0",
2996"placeholder": "",
2997"style": "IPY_MODEL_6ee5d390d87b48888029b917805ac640",
2998"value": " 1/1 [00:00<00:00, 2.84 Batches/s]"
2999}
3000},
3001"93a607e6574f4a0fb3b9b1463a4aad4c": {
3002"model_module": "@jupyter-widgets/base",
3003"model_module_version": "1.2.0",
3004"model_name": "LayoutModel",
3005"state": {
3006"_model_module": "@jupyter-widgets/base",
3007"_model_module_version": "1.2.0",
3008"_model_name": "LayoutModel",
3009"_view_count": null,
3010"_view_module": "@jupyter-widgets/base",
3011"_view_module_version": "1.2.0",
3012"_view_name": "LayoutView",
3013"align_content": null,
3014"align_items": null,
3015"align_self": null,
3016"border": null,
3017"bottom": null,
3018"display": null,
3019"flex": null,
3020"flex_flow": null,
3021"grid_area": null,
3022"grid_auto_columns": null,
3023"grid_auto_flow": null,
3024"grid_auto_rows": null,
3025"grid_column": null,
3026"grid_gap": null,
3027"grid_row": null,
3028"grid_template_areas": null,
3029"grid_template_columns": null,
3030"grid_template_rows": null,
3031"height": null,
3032"justify_content": null,
3033"justify_items": null,
3034"left": null,
3035"margin": null,
3036"max_height": null,
3037"max_width": null,
3038"min_height": null,
3039"min_width": null,
3040"object_fit": null,
3041"object_position": null,
3042"order": null,
3043"overflow": null,
3044"overflow_x": null,
3045"overflow_y": null,
3046"padding": null,
3047"right": null,
3048"top": null,
3049"visibility": null,
3050"width": null
3051}
3052},
3053"95b8b7dcd2ff4152b8a684e5cb878a91": {
3054"model_module": "@jupyter-widgets/controls",
3055"model_module_version": "1.5.0",
3056"model_name": "DescriptionStyleModel",
3057"state": {
3058"_model_module": "@jupyter-widgets/controls",
3059"_model_module_version": "1.5.0",
3060"_model_name": "DescriptionStyleModel",
3061"_view_count": null,
3062"_view_module": "@jupyter-widgets/base",
3063"_view_module_version": "1.2.0",
3064"_view_name": "StyleView",
3065"description_width": ""
3066}
3067},
3068"96e57e14ae6e45bab18b8f940c048507": {
3069"model_module": "@jupyter-widgets/base",
3070"model_module_version": "1.2.0",
3071"model_name": "LayoutModel",
3072"state": {
3073"_model_module": "@jupyter-widgets/base",
3074"_model_module_version": "1.2.0",
3075"_model_name": "LayoutModel",
3076"_view_count": null,
3077"_view_module": "@jupyter-widgets/base",
3078"_view_module_version": "1.2.0",
3079"_view_name": "LayoutView",
3080"align_content": null,
3081"align_items": null,
3082"align_self": null,
3083"border": null,
3084"bottom": null,
3085"display": null,
3086"flex": null,
3087"flex_flow": null,
3088"grid_area": null,
3089"grid_auto_columns": null,
3090"grid_auto_flow": null,
3091"grid_auto_rows": null,
3092"grid_column": null,
3093"grid_gap": null,
3094"grid_row": null,
3095"grid_template_areas": null,
3096"grid_template_columns": null,
3097"grid_template_rows": null,
3098"height": null,
3099"justify_content": null,
3100"justify_items": null,
3101"left": null,
3102"margin": null,
3103"max_height": null,
3104"max_width": null,
3105"min_height": null,
3106"min_width": null,
3107"object_fit": null,
3108"object_position": null,
3109"order": null,
3110"overflow": null,
3111"overflow_x": null,
3112"overflow_y": null,
3113"padding": null,
3114"right": null,
3115"top": null,
3116"visibility": null,
3117"width": null
3118}
3119},
3120"9962218c39cf4d20abc2460278fd6808": {
3121"model_module": "@jupyter-widgets/controls",
3122"model_module_version": "1.5.0",
3123"model_name": "HTMLModel",
3124"state": {
3125"_dom_classes": [],
3126"_model_module": "@jupyter-widgets/controls",
3127"_model_module_version": "1.5.0",
3128"_model_name": "HTMLModel",
3129"_view_count": null,
3130"_view_module": "@jupyter-widgets/controls",
3131"_view_module_version": "1.5.0",
3132"_view_name": "HTMLView",
3133"description": "",
3134"description_tooltip": null,
3135"layout": "IPY_MODEL_633687b1bf3e49b2bbe5c8fcdf21b187",
3136"placeholder": "",
3137"style": "IPY_MODEL_9eb4bff064f7491d8e90bb7ab270d0c2",
3138"value": " 1/1 [00:00<00:00, 32.28it/s]"
3139}
3140},
3141"9989d5d79a3a41fa9bc0431465997611": {
3142"model_module": "@jupyter-widgets/controls",
3143"model_module_version": "1.5.0",
3144"model_name": "FloatProgressModel",
3145"state": {
3146"_dom_classes": [],
3147"_model_module": "@jupyter-widgets/controls",
3148"_model_module_version": "1.5.0",
3149"_model_name": "FloatProgressModel",
3150"_view_count": null,
3151"_view_module": "@jupyter-widgets/controls",
3152"_view_module_version": "1.5.0",
3153"_view_name": "ProgressView",
3154"bar_style": "success",
3155"description": "",
3156"description_tooltip": null,
3157"layout": "IPY_MODEL_333a2e1b38f14049bcd8633fb614dfd1",
3158"max": 1,
3159"min": 0,
3160"orientation": "horizontal",
3161"style": "IPY_MODEL_05e19ba65d5b4df09c6a93551affbf67",
3162"value": 1
3163}
3164},
3165"99945c16563d40a5aa0825a1134a6300": {
3166"model_module": "@jupyter-widgets/base",
3167"model_module_version": "1.2.0",
3168"model_name": "LayoutModel",
3169"state": {
3170"_model_module": "@jupyter-widgets/base",
3171"_model_module_version": "1.2.0",
3172"_model_name": "LayoutModel",
3173"_view_count": null,
3174"_view_module": "@jupyter-widgets/base",
3175"_view_module_version": "1.2.0",
3176"_view_name": "LayoutView",
3177"align_content": null,
3178"align_items": null,
3179"align_self": null,
3180"border": null,
3181"bottom": null,
3182"display": null,
3183"flex": null,
3184"flex_flow": null,
3185"grid_area": null,
3186"grid_auto_columns": null,
3187"grid_auto_flow": null,
3188"grid_auto_rows": null,
3189"grid_column": null,
3190"grid_gap": null,
3191"grid_row": null,
3192"grid_template_areas": null,
3193"grid_template_columns": null,
3194"grid_template_rows": null,
3195"height": null,
3196"justify_content": null,
3197"justify_items": null,
3198"left": null,
3199"margin": null,
3200"max_height": null,
3201"max_width": null,
3202"min_height": null,
3203"min_width": null,
3204"object_fit": null,
3205"object_position": null,
3206"order": null,
3207"overflow": null,
3208"overflow_x": null,
3209"overflow_y": null,
3210"padding": null,
3211"right": null,
3212"top": null,
3213"visibility": null,
3214"width": null
3215}
3216},
3217"9a4a31fda4e64a27ae2c884ad1e9d9c9": {
3218"model_module": "@jupyter-widgets/controls",
3219"model_module_version": "1.5.0",
3220"model_name": "HTMLModel",
3221"state": {
3222"_dom_classes": [],
3223"_model_module": "@jupyter-widgets/controls",
3224"_model_module_version": "1.5.0",
3225"_model_name": "HTMLModel",
3226"_view_count": null,
3227"_view_module": "@jupyter-widgets/controls",
3228"_view_module_version": "1.5.0",
3229"_view_name": "HTMLView",
3230"description": "",
3231"description_tooltip": null,
3232"layout": "IPY_MODEL_ac6c13dab196486d87d249b3f5382e86",
3233"placeholder": "",
3234"style": "IPY_MODEL_d5e111a594d3475dbdff20071822e139",
3235"value": "Inferencing Samples: 100%"
3236}
3237},
3238"9d4376e02eb7424ba13297548867ac44": {
3239"model_module": "@jupyter-widgets/controls",
3240"model_module_version": "1.5.0",
3241"model_name": "FloatProgressModel",
3242"state": {
3243"_dom_classes": [],
3244"_model_module": "@jupyter-widgets/controls",
3245"_model_module_version": "1.5.0",
3246"_model_name": "FloatProgressModel",
3247"_view_count": null,
3248"_view_module": "@jupyter-widgets/controls",
3249"_view_module_version": "1.5.0",
3250"_view_name": "ProgressView",
3251"bar_style": "success",
3252"description": "",
3253"description_tooltip": null,
3254"layout": "IPY_MODEL_170f6a48eb4249e3a44d56878415d94f",
3255"max": 1,
3256"min": 0,
3257"orientation": "horizontal",
3258"style": "IPY_MODEL_55405182fbc24485934754b53eac871b",
3259"value": 1
3260}
3261},
3262"9e52e775dc5640be920eebc454aaa67c": {
3263"model_module": "@jupyter-widgets/base",
3264"model_module_version": "1.2.0",
3265"model_name": "LayoutModel",
3266"state": {
3267"_model_module": "@jupyter-widgets/base",
3268"_model_module_version": "1.2.0",
3269"_model_name": "LayoutModel",
3270"_view_count": null,
3271"_view_module": "@jupyter-widgets/base",
3272"_view_module_version": "1.2.0",
3273"_view_name": "LayoutView",
3274"align_content": null,
3275"align_items": null,
3276"align_self": null,
3277"border": null,
3278"bottom": null,
3279"display": null,
3280"flex": null,
3281"flex_flow": null,
3282"grid_area": null,
3283"grid_auto_columns": null,
3284"grid_auto_flow": null,
3285"grid_auto_rows": null,
3286"grid_column": null,
3287"grid_gap": null,
3288"grid_row": null,
3289"grid_template_areas": null,
3290"grid_template_columns": null,
3291"grid_template_rows": null,
3292"height": null,
3293"justify_content": null,
3294"justify_items": null,
3295"left": null,
3296"margin": null,
3297"max_height": null,
3298"max_width": null,
3299"min_height": null,
3300"min_width": null,
3301"object_fit": null,
3302"object_position": null,
3303"order": null,
3304"overflow": null,
3305"overflow_x": null,
3306"overflow_y": null,
3307"padding": null,
3308"right": null,
3309"top": null,
3310"visibility": null,
3311"width": null
3312}
3313},
3314"9eb4bff064f7491d8e90bb7ab270d0c2": {
3315"model_module": "@jupyter-widgets/controls",
3316"model_module_version": "1.5.0",
3317"model_name": "DescriptionStyleModel",
3318"state": {
3319"_model_module": "@jupyter-widgets/controls",
3320"_model_module_version": "1.5.0",
3321"_model_name": "DescriptionStyleModel",
3322"_view_count": null,
3323"_view_module": "@jupyter-widgets/base",
3324"_view_module_version": "1.2.0",
3325"_view_name": "StyleView",
3326"description_width": ""
3327}
3328},
3329"a0d604c26d75411b870a729695ea8341": {
3330"model_module": "@jupyter-widgets/controls",
3331"model_module_version": "1.5.0",
3332"model_name": "ProgressStyleModel",
3333"state": {
3334"_model_module": "@jupyter-widgets/controls",
3335"_model_module_version": "1.5.0",
3336"_model_name": "ProgressStyleModel",
3337"_view_count": null,
3338"_view_module": "@jupyter-widgets/base",
3339"_view_module_version": "1.2.0",
3340"_view_name": "StyleView",
3341"bar_color": null,
3342"description_width": ""
3343}
3344},
3345"a1022f00dda143778c1a1ebc80f7e878": {
3346"model_module": "@jupyter-widgets/controls",
3347"model_module_version": "1.5.0",
3348"model_name": "ProgressStyleModel",
3349"state": {
3350"_model_module": "@jupyter-widgets/controls",
3351"_model_module_version": "1.5.0",
3352"_model_name": "ProgressStyleModel",
3353"_view_count": null,
3354"_view_module": "@jupyter-widgets/base",
3355"_view_module_version": "1.2.0",
3356"_view_name": "StyleView",
3357"bar_color": null,
3358"description_width": ""
3359}
3360},
3361"a20d8628bdb5486a8ccab95660a986c3": {
3362"model_module": "@jupyter-widgets/controls",
3363"model_module_version": "1.5.0",
3364"model_name": "FloatProgressModel",
3365"state": {
3366"_dom_classes": [],
3367"_model_module": "@jupyter-widgets/controls",
3368"_model_module_version": "1.5.0",
3369"_model_name": "FloatProgressModel",
3370"_view_count": null,
3371"_view_module": "@jupyter-widgets/controls",
3372"_view_module_version": "1.5.0",
3373"_view_name": "ProgressView",
3374"bar_style": "success",
3375"description": "",
3376"description_tooltip": null,
3377"layout": "IPY_MODEL_4c514834225147eab4ba48b7ece93ecb",
3378"max": 1,
3379"min": 0,
3380"orientation": "horizontal",
3381"style": "IPY_MODEL_c70f352bec2f441990a5e538cf644d6a",
3382"value": 1
3383}
3384},
3385"a53cdfb5315a42beb72569956916cdfa": {
3386"model_module": "@jupyter-widgets/controls",
3387"model_module_version": "1.5.0",
3388"model_name": "HTMLModel",
3389"state": {
3390"_dom_classes": [],
3391"_model_module": "@jupyter-widgets/controls",
3392"_model_module_version": "1.5.0",
3393"_model_name": "HTMLModel",
3394"_view_count": null,
3395"_view_module": "@jupyter-widgets/controls",
3396"_view_module_version": "1.5.0",
3397"_view_name": "HTMLView",
3398"description": "",
3399"description_tooltip": null,
3400"layout": "IPY_MODEL_96e57e14ae6e45bab18b8f940c048507",
3401"placeholder": "",
3402"style": "IPY_MODEL_f6bac06384a84aea8a470bf612e90d2a",
3403"value": "Inferencing Samples: 100%"
3404}
3405},
3406"a9e34231df9548e584c81ea89b814ee6": {
3407"model_module": "@jupyter-widgets/controls",
3408"model_module_version": "1.5.0",
3409"model_name": "HTMLModel",
3410"state": {
3411"_dom_classes": [],
3412"_model_module": "@jupyter-widgets/controls",
3413"_model_module_version": "1.5.0",
3414"_model_name": "HTMLModel",
3415"_view_count": null,
3416"_view_module": "@jupyter-widgets/controls",
3417"_view_module_version": "1.5.0",
3418"_view_name": "HTMLView",
3419"description": "",
3420"description_tooltip": null,
3421"layout": "IPY_MODEL_c78aa680ada54f29bc8bbe8ce94dfe31",
3422"placeholder": "",
3423"style": "IPY_MODEL_0e3b35c207e34b738c179ed6de3fb906",
3424"value": "Batches: 100%"
3425}
3426},
3427"ac6c13dab196486d87d249b3f5382e86": {
3428"model_module": "@jupyter-widgets/base",
3429"model_module_version": "1.2.0",
3430"model_name": "LayoutModel",
3431"state": {
3432"_model_module": "@jupyter-widgets/base",
3433"_model_module_version": "1.2.0",
3434"_model_name": "LayoutModel",
3435"_view_count": null,
3436"_view_module": "@jupyter-widgets/base",
3437"_view_module_version": "1.2.0",
3438"_view_name": "LayoutView",
3439"align_content": null,
3440"align_items": null,
3441"align_self": null,
3442"border": null,
3443"bottom": null,
3444"display": null,
3445"flex": null,
3446"flex_flow": null,
3447"grid_area": null,
3448"grid_auto_columns": null,
3449"grid_auto_flow": null,
3450"grid_auto_rows": null,
3451"grid_column": null,
3452"grid_gap": null,
3453"grid_row": null,
3454"grid_template_areas": null,
3455"grid_template_columns": null,
3456"grid_template_rows": null,
3457"height": null,
3458"justify_content": null,
3459"justify_items": null,
3460"left": null,
3461"margin": null,
3462"max_height": null,
3463"max_width": null,
3464"min_height": null,
3465"min_width": null,
3466"object_fit": null,
3467"object_position": null,
3468"order": null,
3469"overflow": null,
3470"overflow_x": null,
3471"overflow_y": null,
3472"padding": null,
3473"right": null,
3474"top": null,
3475"visibility": null,
3476"width": null
3477}
3478},
3479"ad54965c74384ab283e7c638d254d763": {
3480"model_module": "@jupyter-widgets/base",
3481"model_module_version": "1.2.0",
3482"model_name": "LayoutModel",
3483"state": {
3484"_model_module": "@jupyter-widgets/base",
3485"_model_module_version": "1.2.0",
3486"_model_name": "LayoutModel",
3487"_view_count": null,
3488"_view_module": "@jupyter-widgets/base",
3489"_view_module_version": "1.2.0",
3490"_view_name": "LayoutView",
3491"align_content": null,
3492"align_items": null,
3493"align_self": null,
3494"border": null,
3495"bottom": null,
3496"display": null,
3497"flex": null,
3498"flex_flow": null,
3499"grid_area": null,
3500"grid_auto_columns": null,
3501"grid_auto_flow": null,
3502"grid_auto_rows": null,
3503"grid_column": null,
3504"grid_gap": null,
3505"grid_row": null,
3506"grid_template_areas": null,
3507"grid_template_columns": null,
3508"grid_template_rows": null,
3509"height": null,
3510"justify_content": null,
3511"justify_items": null,
3512"left": null,
3513"margin": null,
3514"max_height": null,
3515"max_width": null,
3516"min_height": null,
3517"min_width": null,
3518"object_fit": null,
3519"object_position": null,
3520"order": null,
3521"overflow": null,
3522"overflow_x": null,
3523"overflow_y": null,
3524"padding": null,
3525"right": null,
3526"top": null,
3527"visibility": null,
3528"width": null
3529}
3530},
3531"b096be01767543418953eae9bc148cb1": {
3532"model_module": "@jupyter-widgets/controls",
3533"model_module_version": "1.5.0",
3534"model_name": "DescriptionStyleModel",
3535"state": {
3536"_model_module": "@jupyter-widgets/controls",
3537"_model_module_version": "1.5.0",
3538"_model_name": "DescriptionStyleModel",
3539"_view_count": null,
3540"_view_module": "@jupyter-widgets/base",
3541"_view_module_version": "1.2.0",
3542"_view_name": "StyleView",
3543"description_width": ""
3544}
3545},
3546"b20df6374f924e7e896d58e93fd45ae4": {
3547"model_module": "@jupyter-widgets/controls",
3548"model_module_version": "1.5.0",
3549"model_name": "HTMLModel",
3550"state": {
3551"_dom_classes": [],
3552"_model_module": "@jupyter-widgets/controls",
3553"_model_module_version": "1.5.0",
3554"_model_name": "HTMLModel",
3555"_view_count": null,
3556"_view_module": "@jupyter-widgets/controls",
3557"_view_module_version": "1.5.0",
3558"_view_name": "HTMLView",
3559"description": "",
3560"description_tooltip": null,
3561"layout": "IPY_MODEL_9258bf70f05f4f3d96621a5c5a2a8a75",
3562"placeholder": "",
3563"style": "IPY_MODEL_38e8dfef6cbf4f2b82471b74d3814177",
3564"value": " 1/1 [00:00<00:00, 2.90 Batches/s]"
3565}
3566},
3567"bbfb9e1799aa4580aef7cbf88d604d84": {
3568"model_module": "@jupyter-widgets/base",
3569"model_module_version": "1.2.0",
3570"model_name": "LayoutModel",
3571"state": {
3572"_model_module": "@jupyter-widgets/base",
3573"_model_module_version": "1.2.0",
3574"_model_name": "LayoutModel",
3575"_view_count": null,
3576"_view_module": "@jupyter-widgets/base",
3577"_view_module_version": "1.2.0",
3578"_view_name": "LayoutView",
3579"align_content": null,
3580"align_items": null,
3581"align_self": null,
3582"border": null,
3583"bottom": null,
3584"display": null,
3585"flex": null,
3586"flex_flow": null,
3587"grid_area": null,
3588"grid_auto_columns": null,
3589"grid_auto_flow": null,
3590"grid_auto_rows": null,
3591"grid_column": null,
3592"grid_gap": null,
3593"grid_row": null,
3594"grid_template_areas": null,
3595"grid_template_columns": null,
3596"grid_template_rows": null,
3597"height": null,
3598"justify_content": null,
3599"justify_items": null,
3600"left": null,
3601"margin": null,
3602"max_height": null,
3603"max_width": null,
3604"min_height": null,
3605"min_width": null,
3606"object_fit": null,
3607"object_position": null,
3608"order": null,
3609"overflow": null,
3610"overflow_x": null,
3611"overflow_y": null,
3612"padding": null,
3613"right": null,
3614"top": null,
3615"visibility": null,
3616"width": null
3617}
3618},
3619"bd2fb1c05642482bbed1e4f4e2824f80": {
3620"model_module": "@jupyter-widgets/base",
3621"model_module_version": "1.2.0",
3622"model_name": "LayoutModel",
3623"state": {
3624"_model_module": "@jupyter-widgets/base",
3625"_model_module_version": "1.2.0",
3626"_model_name": "LayoutModel",
3627"_view_count": null,
3628"_view_module": "@jupyter-widgets/base",
3629"_view_module_version": "1.2.0",
3630"_view_name": "LayoutView",
3631"align_content": null,
3632"align_items": null,
3633"align_self": null,
3634"border": null,
3635"bottom": null,
3636"display": null,
3637"flex": null,
3638"flex_flow": null,
3639"grid_area": null,
3640"grid_auto_columns": null,
3641"grid_auto_flow": null,
3642"grid_auto_rows": null,
3643"grid_column": null,
3644"grid_gap": null,
3645"grid_row": null,
3646"grid_template_areas": null,
3647"grid_template_columns": null,
3648"grid_template_rows": null,
3649"height": null,
3650"justify_content": null,
3651"justify_items": null,
3652"left": null,
3653"margin": null,
3654"max_height": null,
3655"max_width": null,
3656"min_height": null,
3657"min_width": null,
3658"object_fit": null,
3659"object_position": null,
3660"order": null,
3661"overflow": null,
3662"overflow_x": null,
3663"overflow_y": null,
3664"padding": null,
3665"right": null,
3666"top": null,
3667"visibility": null,
3668"width": null
3669}
3670},
3671"be6dd7e2f43748d490deda9bab833ce4": {
3672"model_module": "@jupyter-widgets/base",
3673"model_module_version": "1.2.0",
3674"model_name": "LayoutModel",
3675"state": {
3676"_model_module": "@jupyter-widgets/base",
3677"_model_module_version": "1.2.0",
3678"_model_name": "LayoutModel",
3679"_view_count": null,
3680"_view_module": "@jupyter-widgets/base",
3681"_view_module_version": "1.2.0",
3682"_view_name": "LayoutView",
3683"align_content": null,
3684"align_items": null,
3685"align_self": null,
3686"border": null,
3687"bottom": null,
3688"display": null,
3689"flex": null,
3690"flex_flow": null,
3691"grid_area": null,
3692"grid_auto_columns": null,
3693"grid_auto_flow": null,
3694"grid_auto_rows": null,
3695"grid_column": null,
3696"grid_gap": null,
3697"grid_row": null,
3698"grid_template_areas": null,
3699"grid_template_columns": null,
3700"grid_template_rows": null,
3701"height": null,
3702"justify_content": null,
3703"justify_items": null,
3704"left": null,
3705"margin": null,
3706"max_height": null,
3707"max_width": null,
3708"min_height": null,
3709"min_width": null,
3710"object_fit": null,
3711"object_position": null,
3712"order": null,
3713"overflow": null,
3714"overflow_x": null,
3715"overflow_y": null,
3716"padding": null,
3717"right": null,
3718"top": null,
3719"visibility": null,
3720"width": null
3721}
3722},
3723"bed20d405095472e8b5166f1d9d1b041": {
3724"model_module": "@jupyter-widgets/controls",
3725"model_module_version": "1.5.0",
3726"model_name": "HBoxModel",
3727"state": {
3728"_dom_classes": [],
3729"_model_module": "@jupyter-widgets/controls",
3730"_model_module_version": "1.5.0",
3731"_model_name": "HBoxModel",
3732"_view_count": null,
3733"_view_module": "@jupyter-widgets/controls",
3734"_view_module_version": "1.5.0",
3735"_view_name": "HBoxView",
3736"box_style": "",
3737"children": [
3738"IPY_MODEL_a53cdfb5315a42beb72569956916cdfa",
3739"IPY_MODEL_f12d6748a4bc478c92d8e8ed8730cce2",
3740"IPY_MODEL_b20df6374f924e7e896d58e93fd45ae4"
3741],
3742"layout": "IPY_MODEL_8780553fdded4f0b906e0ce670a1657e"
3743}
3744},
3745"c050d37c2f4642b0925abd9e2eb06d99": {
3746"model_module": "@jupyter-widgets/base",
3747"model_module_version": "1.2.0",
3748"model_name": "LayoutModel",
3749"state": {
3750"_model_module": "@jupyter-widgets/base",
3751"_model_module_version": "1.2.0",
3752"_model_name": "LayoutModel",
3753"_view_count": null,
3754"_view_module": "@jupyter-widgets/base",
3755"_view_module_version": "1.2.0",
3756"_view_name": "LayoutView",
3757"align_content": null,
3758"align_items": null,
3759"align_self": null,
3760"border": null,
3761"bottom": null,
3762"display": null,
3763"flex": null,
3764"flex_flow": null,
3765"grid_area": null,
3766"grid_auto_columns": null,
3767"grid_auto_flow": null,
3768"grid_auto_rows": null,
3769"grid_column": null,
3770"grid_gap": null,
3771"grid_row": null,
3772"grid_template_areas": null,
3773"grid_template_columns": null,
3774"grid_template_rows": null,
3775"height": null,
3776"justify_content": null,
3777"justify_items": null,
3778"left": null,
3779"margin": null,
3780"max_height": null,
3781"max_width": null,
3782"min_height": null,
3783"min_width": null,
3784"object_fit": null,
3785"object_position": null,
3786"order": null,
3787"overflow": null,
3788"overflow_x": null,
3789"overflow_y": null,
3790"padding": null,
3791"right": null,
3792"top": null,
3793"visibility": null,
3794"width": null
3795}
3796},
3797"c0b9068361404c5ab60bb7d4b3dca265": {
3798"model_module": "@jupyter-widgets/base",
3799"model_module_version": "1.2.0",
3800"model_name": "LayoutModel",
3801"state": {
3802"_model_module": "@jupyter-widgets/base",
3803"_model_module_version": "1.2.0",
3804"_model_name": "LayoutModel",
3805"_view_count": null,
3806"_view_module": "@jupyter-widgets/base",
3807"_view_module_version": "1.2.0",
3808"_view_name": "LayoutView",
3809"align_content": null,
3810"align_items": null,
3811"align_self": null,
3812"border": null,
3813"bottom": null,
3814"display": null,
3815"flex": null,
3816"flex_flow": null,
3817"grid_area": null,
3818"grid_auto_columns": null,
3819"grid_auto_flow": null,
3820"grid_auto_rows": null,
3821"grid_column": null,
3822"grid_gap": null,
3823"grid_row": null,
3824"grid_template_areas": null,
3825"grid_template_columns": null,
3826"grid_template_rows": null,
3827"height": null,
3828"justify_content": null,
3829"justify_items": null,
3830"left": null,
3831"margin": null,
3832"max_height": null,
3833"max_width": null,
3834"min_height": null,
3835"min_width": null,
3836"object_fit": null,
3837"object_position": null,
3838"order": null,
3839"overflow": null,
3840"overflow_x": null,
3841"overflow_y": null,
3842"padding": null,
3843"right": null,
3844"top": null,
3845"visibility": null,
3846"width": null
3847}
3848},
3849"c0cd6e5f853b4ee0858d3b4c53a102ee": {
3850"model_module": "@jupyter-widgets/base",
3851"model_module_version": "1.2.0",
3852"model_name": "LayoutModel",
3853"state": {
3854"_model_module": "@jupyter-widgets/base",
3855"_model_module_version": "1.2.0",
3856"_model_name": "LayoutModel",
3857"_view_count": null,
3858"_view_module": "@jupyter-widgets/base",
3859"_view_module_version": "1.2.0",
3860"_view_name": "LayoutView",
3861"align_content": null,
3862"align_items": null,
3863"align_self": null,
3864"border": null,
3865"bottom": null,
3866"display": null,
3867"flex": null,
3868"flex_flow": null,
3869"grid_area": null,
3870"grid_auto_columns": null,
3871"grid_auto_flow": null,
3872"grid_auto_rows": null,
3873"grid_column": null,
3874"grid_gap": null,
3875"grid_row": null,
3876"grid_template_areas": null,
3877"grid_template_columns": null,
3878"grid_template_rows": null,
3879"height": null,
3880"justify_content": null,
3881"justify_items": null,
3882"left": null,
3883"margin": null,
3884"max_height": null,
3885"max_width": null,
3886"min_height": null,
3887"min_width": null,
3888"object_fit": null,
3889"object_position": null,
3890"order": null,
3891"overflow": null,
3892"overflow_x": null,
3893"overflow_y": null,
3894"padding": null,
3895"right": null,
3896"top": null,
3897"visibility": null,
3898"width": null
3899}
3900},
3901"c321ff97706d465193a2b3dfcb107c1c": {
3902"model_module": "@jupyter-widgets/controls",
3903"model_module_version": "1.5.0",
3904"model_name": "HTMLModel",
3905"state": {
3906"_dom_classes": [],
3907"_model_module": "@jupyter-widgets/controls",
3908"_model_module_version": "1.5.0",
3909"_model_name": "HTMLModel",
3910"_view_count": null,
3911"_view_module": "@jupyter-widgets/controls",
3912"_view_module_version": "1.5.0",
3913"_view_name": "HTMLView",
3914"description": "",
3915"description_tooltip": null,
3916"layout": "IPY_MODEL_1816d2ee14014fe7b76402ceac0cfc67",
3917"placeholder": "",
3918"style": "IPY_MODEL_3298a7b7d5d14f198695f0769e527b60",
3919"value": "Inferencing Samples: 100%"
3920}
3921},
3922"c4539733aed34c7b940de5da7edfa82a": {
3923"model_module": "@jupyter-widgets/base",
3924"model_module_version": "1.2.0",
3925"model_name": "LayoutModel",
3926"state": {
3927"_model_module": "@jupyter-widgets/base",
3928"_model_module_version": "1.2.0",
3929"_model_name": "LayoutModel",
3930"_view_count": null,
3931"_view_module": "@jupyter-widgets/base",
3932"_view_module_version": "1.2.0",
3933"_view_name": "LayoutView",
3934"align_content": null,
3935"align_items": null,
3936"align_self": null,
3937"border": null,
3938"bottom": null,
3939"display": null,
3940"flex": null,
3941"flex_flow": null,
3942"grid_area": null,
3943"grid_auto_columns": null,
3944"grid_auto_flow": null,
3945"grid_auto_rows": null,
3946"grid_column": null,
3947"grid_gap": null,
3948"grid_row": null,
3949"grid_template_areas": null,
3950"grid_template_columns": null,
3951"grid_template_rows": null,
3952"height": null,
3953"justify_content": null,
3954"justify_items": null,
3955"left": null,
3956"margin": null,
3957"max_height": null,
3958"max_width": null,
3959"min_height": null,
3960"min_width": null,
3961"object_fit": null,
3962"object_position": null,
3963"order": null,
3964"overflow": null,
3965"overflow_x": null,
3966"overflow_y": null,
3967"padding": null,
3968"right": null,
3969"top": null,
3970"visibility": null,
3971"width": null
3972}
3973},
3974"c70f352bec2f441990a5e538cf644d6a": {
3975"model_module": "@jupyter-widgets/controls",
3976"model_module_version": "1.5.0",
3977"model_name": "ProgressStyleModel",
3978"state": {
3979"_model_module": "@jupyter-widgets/controls",
3980"_model_module_version": "1.5.0",
3981"_model_name": "ProgressStyleModel",
3982"_view_count": null,
3983"_view_module": "@jupyter-widgets/base",
3984"_view_module_version": "1.2.0",
3985"_view_name": "StyleView",
3986"bar_color": null,
3987"description_width": ""
3988}
3989},
3990"c72dbeb5557b4ac7bc46c957bab2485f": {
3991"model_module": "@jupyter-widgets/base",
3992"model_module_version": "1.2.0",
3993"model_name": "LayoutModel",
3994"state": {
3995"_model_module": "@jupyter-widgets/base",
3996"_model_module_version": "1.2.0",
3997"_model_name": "LayoutModel",
3998"_view_count": null,
3999"_view_module": "@jupyter-widgets/base",
4000"_view_module_version": "1.2.0",
4001"_view_name": "LayoutView",
4002"align_content": null,
4003"align_items": null,
4004"align_self": null,
4005"border": null,
4006"bottom": null,
4007"display": null,
4008"flex": null,
4009"flex_flow": null,
4010"grid_area": null,
4011"grid_auto_columns": null,
4012"grid_auto_flow": null,
4013"grid_auto_rows": null,
4014"grid_column": null,
4015"grid_gap": null,
4016"grid_row": null,
4017"grid_template_areas": null,
4018"grid_template_columns": null,
4019"grid_template_rows": null,
4020"height": null,
4021"justify_content": null,
4022"justify_items": null,
4023"left": null,
4024"margin": null,
4025"max_height": null,
4026"max_width": null,
4027"min_height": null,
4028"min_width": null,
4029"object_fit": null,
4030"object_position": null,
4031"order": null,
4032"overflow": null,
4033"overflow_x": null,
4034"overflow_y": null,
4035"padding": null,
4036"right": null,
4037"top": null,
4038"visibility": null,
4039"width": null
4040}
4041},
4042"c7673d0c64df414981cfc7246a0e01e6": {
4043"model_module": "@jupyter-widgets/base",
4044"model_module_version": "1.2.0",
4045"model_name": "LayoutModel",
4046"state": {
4047"_model_module": "@jupyter-widgets/base",
4048"_model_module_version": "1.2.0",
4049"_model_name": "LayoutModel",
4050"_view_count": null,
4051"_view_module": "@jupyter-widgets/base",
4052"_view_module_version": "1.2.0",
4053"_view_name": "LayoutView",
4054"align_content": null,
4055"align_items": null,
4056"align_self": null,
4057"border": null,
4058"bottom": null,
4059"display": null,
4060"flex": null,
4061"flex_flow": null,
4062"grid_area": null,
4063"grid_auto_columns": null,
4064"grid_auto_flow": null,
4065"grid_auto_rows": null,
4066"grid_column": null,
4067"grid_gap": null,
4068"grid_row": null,
4069"grid_template_areas": null,
4070"grid_template_columns": null,
4071"grid_template_rows": null,
4072"height": null,
4073"justify_content": null,
4074"justify_items": null,
4075"left": null,
4076"margin": null,
4077"max_height": null,
4078"max_width": null,
4079"min_height": null,
4080"min_width": null,
4081"object_fit": null,
4082"object_position": null,
4083"order": null,
4084"overflow": null,
4085"overflow_x": null,
4086"overflow_y": null,
4087"padding": null,
4088"right": null,
4089"top": null,
4090"visibility": null,
4091"width": null
4092}
4093},
4094"c78aa680ada54f29bc8bbe8ce94dfe31": {
4095"model_module": "@jupyter-widgets/base",
4096"model_module_version": "1.2.0",
4097"model_name": "LayoutModel",
4098"state": {
4099"_model_module": "@jupyter-widgets/base",
4100"_model_module_version": "1.2.0",
4101"_model_name": "LayoutModel",
4102"_view_count": null,
4103"_view_module": "@jupyter-widgets/base",
4104"_view_module_version": "1.2.0",
4105"_view_name": "LayoutView",
4106"align_content": null,
4107"align_items": null,
4108"align_self": null,
4109"border": null,
4110"bottom": null,
4111"display": null,
4112"flex": null,
4113"flex_flow": null,
4114"grid_area": null,
4115"grid_auto_columns": null,
4116"grid_auto_flow": null,
4117"grid_auto_rows": null,
4118"grid_column": null,
4119"grid_gap": null,
4120"grid_row": null,
4121"grid_template_areas": null,
4122"grid_template_columns": null,
4123"grid_template_rows": null,
4124"height": null,
4125"justify_content": null,
4126"justify_items": null,
4127"left": null,
4128"margin": null,
4129"max_height": null,
4130"max_width": null,
4131"min_height": null,
4132"min_width": null,
4133"object_fit": null,
4134"object_position": null,
4135"order": null,
4136"overflow": null,
4137"overflow_x": null,
4138"overflow_y": null,
4139"padding": null,
4140"right": null,
4141"top": null,
4142"visibility": null,
4143"width": null
4144}
4145},
4146"c7d62339c034489b8dd9ade762892ff8": {
4147"model_module": "@jupyter-widgets/controls",
4148"model_module_version": "1.5.0",
4149"model_name": "HTMLModel",
4150"state": {
4151"_dom_classes": [],
4152"_model_module": "@jupyter-widgets/controls",
4153"_model_module_version": "1.5.0",
4154"_model_name": "HTMLModel",
4155"_view_count": null,
4156"_view_module": "@jupyter-widgets/controls",
4157"_view_module_version": "1.5.0",
4158"_view_name": "HTMLView",
4159"description": "",
4160"description_tooltip": null,
4161"layout": "IPY_MODEL_d861455dc8344b1fbb03c966e8a803ad",
4162"placeholder": "",
4163"style": "IPY_MODEL_156b4ec959084fbdbcdfb9f7a12ece5f",
4164"value": " 1/1 [00:00<00:00, 2.87 Batches/s]"
4165}
4166},
4167"c88f10e17ab94da3852ae0f8ac7ff7db": {
4168"model_module": "@jupyter-widgets/controls",
4169"model_module_version": "1.5.0",
4170"model_name": "FloatProgressModel",
4171"state": {
4172"_dom_classes": [],
4173"_model_module": "@jupyter-widgets/controls",
4174"_model_module_version": "1.5.0",
4175"_model_name": "FloatProgressModel",
4176"_view_count": null,
4177"_view_module": "@jupyter-widgets/controls",
4178"_view_module_version": "1.5.0",
4179"_view_name": "ProgressView",
4180"bar_style": "success",
4181"description": "",
4182"description_tooltip": null,
4183"layout": "IPY_MODEL_eaa5a7cf338f4c4faf1f4221684f6fa1",
4184"max": 1,
4185"min": 0,
4186"orientation": "horizontal",
4187"style": "IPY_MODEL_2d800606643942e0b0616c1655142793",
4188"value": 1
4189}
4190},
4191"ca94bc5b7543455ebe388c4c934b7664": {
4192"model_module": "@jupyter-widgets/controls",
4193"model_module_version": "1.5.0",
4194"model_name": "HTMLModel",
4195"state": {
4196"_dom_classes": [],
4197"_model_module": "@jupyter-widgets/controls",
4198"_model_module_version": "1.5.0",
4199"_model_name": "HTMLModel",
4200"_view_count": null,
4201"_view_module": "@jupyter-widgets/controls",
4202"_view_module_version": "1.5.0",
4203"_view_name": "HTMLView",
4204"description": "",
4205"description_tooltip": null,
4206"layout": "IPY_MODEL_ef3fd3ff0c1342958346746f939aa48a",
4207"placeholder": "",
4208"style": "IPY_MODEL_dfe261365e144254ae00ea321579b860",
4209"value": "Inferencing Samples: 100%"
4210}
4211},
4212"cc2586d0b77349b2b68a0b05e410f95f": {
4213"model_module": "@jupyter-widgets/base",
4214"model_module_version": "1.2.0",
4215"model_name": "LayoutModel",
4216"state": {
4217"_model_module": "@jupyter-widgets/base",
4218"_model_module_version": "1.2.0",
4219"_model_name": "LayoutModel",
4220"_view_count": null,
4221"_view_module": "@jupyter-widgets/base",
4222"_view_module_version": "1.2.0",
4223"_view_name": "LayoutView",
4224"align_content": null,
4225"align_items": null,
4226"align_self": null,
4227"border": null,
4228"bottom": null,
4229"display": null,
4230"flex": null,
4231"flex_flow": null,
4232"grid_area": null,
4233"grid_auto_columns": null,
4234"grid_auto_flow": null,
4235"grid_auto_rows": null,
4236"grid_column": null,
4237"grid_gap": null,
4238"grid_row": null,
4239"grid_template_areas": null,
4240"grid_template_columns": null,
4241"grid_template_rows": null,
4242"height": null,
4243"justify_content": null,
4244"justify_items": null,
4245"left": null,
4246"margin": null,
4247"max_height": null,
4248"max_width": null,
4249"min_height": null,
4250"min_width": null,
4251"object_fit": null,
4252"object_position": null,
4253"order": null,
4254"overflow": null,
4255"overflow_x": null,
4256"overflow_y": null,
4257"padding": null,
4258"right": null,
4259"top": null,
4260"visibility": null,
4261"width": null
4262}
4263},
4264"ce171e8d143d48569f426f3870ea977a": {
4265"model_module": "@jupyter-widgets/base",
4266"model_module_version": "1.2.0",
4267"model_name": "LayoutModel",
4268"state": {
4269"_model_module": "@jupyter-widgets/base",
4270"_model_module_version": "1.2.0",
4271"_model_name": "LayoutModel",
4272"_view_count": null,
4273"_view_module": "@jupyter-widgets/base",
4274"_view_module_version": "1.2.0",
4275"_view_name": "LayoutView",
4276"align_content": null,
4277"align_items": null,
4278"align_self": null,
4279"border": null,
4280"bottom": null,
4281"display": null,
4282"flex": null,
4283"flex_flow": null,
4284"grid_area": null,
4285"grid_auto_columns": null,
4286"grid_auto_flow": null,
4287"grid_auto_rows": null,
4288"grid_column": null,
4289"grid_gap": null,
4290"grid_row": null,
4291"grid_template_areas": null,
4292"grid_template_columns": null,
4293"grid_template_rows": null,
4294"height": null,
4295"justify_content": null,
4296"justify_items": null,
4297"left": null,
4298"margin": null,
4299"max_height": null,
4300"max_width": null,
4301"min_height": null,
4302"min_width": null,
4303"object_fit": null,
4304"object_position": null,
4305"order": null,
4306"overflow": null,
4307"overflow_x": null,
4308"overflow_y": null,
4309"padding": null,
4310"right": null,
4311"top": null,
4312"visibility": null,
4313"width": null
4314}
4315},
4316"cf10491e88374c679c94b757ef207144": {
4317"model_module": "@jupyter-widgets/controls",
4318"model_module_version": "1.5.0",
4319"model_name": "ProgressStyleModel",
4320"state": {
4321"_model_module": "@jupyter-widgets/controls",
4322"_model_module_version": "1.5.0",
4323"_model_name": "ProgressStyleModel",
4324"_view_count": null,
4325"_view_module": "@jupyter-widgets/base",
4326"_view_module_version": "1.2.0",
4327"_view_name": "StyleView",
4328"bar_color": null,
4329"description_width": ""
4330}
4331},
4332"d3d1971a3e3748c9ac7483faafbee1a0": {
4333"model_module": "@jupyter-widgets/base",
4334"model_module_version": "1.2.0",
4335"model_name": "LayoutModel",
4336"state": {
4337"_model_module": "@jupyter-widgets/base",
4338"_model_module_version": "1.2.0",
4339"_model_name": "LayoutModel",
4340"_view_count": null,
4341"_view_module": "@jupyter-widgets/base",
4342"_view_module_version": "1.2.0",
4343"_view_name": "LayoutView",
4344"align_content": null,
4345"align_items": null,
4346"align_self": null,
4347"border": null,
4348"bottom": null,
4349"display": null,
4350"flex": null,
4351"flex_flow": null,
4352"grid_area": null,
4353"grid_auto_columns": null,
4354"grid_auto_flow": null,
4355"grid_auto_rows": null,
4356"grid_column": null,
4357"grid_gap": null,
4358"grid_row": null,
4359"grid_template_areas": null,
4360"grid_template_columns": null,
4361"grid_template_rows": null,
4362"height": null,
4363"justify_content": null,
4364"justify_items": null,
4365"left": null,
4366"margin": null,
4367"max_height": null,
4368"max_width": null,
4369"min_height": null,
4370"min_width": null,
4371"object_fit": null,
4372"object_position": null,
4373"order": null,
4374"overflow": null,
4375"overflow_x": null,
4376"overflow_y": null,
4377"padding": null,
4378"right": null,
4379"top": null,
4380"visibility": null,
4381"width": null
4382}
4383},
4384"d5630f9ff5f546c9871f5f2b76fb305a": {
4385"model_module": "@jupyter-widgets/base",
4386"model_module_version": "1.2.0",
4387"model_name": "LayoutModel",
4388"state": {
4389"_model_module": "@jupyter-widgets/base",
4390"_model_module_version": "1.2.0",
4391"_model_name": "LayoutModel",
4392"_view_count": null,
4393"_view_module": "@jupyter-widgets/base",
4394"_view_module_version": "1.2.0",
4395"_view_name": "LayoutView",
4396"align_content": null,
4397"align_items": null,
4398"align_self": null,
4399"border": null,
4400"bottom": null,
4401"display": null,
4402"flex": null,
4403"flex_flow": null,
4404"grid_area": null,
4405"grid_auto_columns": null,
4406"grid_auto_flow": null,
4407"grid_auto_rows": null,
4408"grid_column": null,
4409"grid_gap": null,
4410"grid_row": null,
4411"grid_template_areas": null,
4412"grid_template_columns": null,
4413"grid_template_rows": null,
4414"height": null,
4415"justify_content": null,
4416"justify_items": null,
4417"left": null,
4418"margin": null,
4419"max_height": null,
4420"max_width": null,
4421"min_height": null,
4422"min_width": null,
4423"object_fit": null,
4424"object_position": null,
4425"order": null,
4426"overflow": null,
4427"overflow_x": null,
4428"overflow_y": null,
4429"padding": null,
4430"right": null,
4431"top": null,
4432"visibility": null,
4433"width": null
4434}
4435},
4436"d5c66b0a453f4a03954fabfd85187e83": {
4437"model_module": "@jupyter-widgets/controls",
4438"model_module_version": "1.5.0",
4439"model_name": "HTMLModel",
4440"state": {
4441"_dom_classes": [],
4442"_model_module": "@jupyter-widgets/controls",
4443"_model_module_version": "1.5.0",
4444"_model_name": "HTMLModel",
4445"_view_count": null,
4446"_view_module": "@jupyter-widgets/controls",
4447"_view_module_version": "1.5.0",
4448"_view_name": "HTMLView",
4449"description": "",
4450"description_tooltip": null,
4451"layout": "IPY_MODEL_99945c16563d40a5aa0825a1134a6300",
4452"placeholder": "",
4453"style": "IPY_MODEL_f39c9f27960e4ff99bd7aef02f14ffd2",
4454"value": " 1/1 [00:00<00:00, 2.14 Batches/s]"
4455}
4456},
4457"d5e111a594d3475dbdff20071822e139": {
4458"model_module": "@jupyter-widgets/controls",
4459"model_module_version": "1.5.0",
4460"model_name": "DescriptionStyleModel",
4461"state": {
4462"_model_module": "@jupyter-widgets/controls",
4463"_model_module_version": "1.5.0",
4464"_model_name": "DescriptionStyleModel",
4465"_view_count": null,
4466"_view_module": "@jupyter-widgets/base",
4467"_view_module_version": "1.2.0",
4468"_view_name": "StyleView",
4469"description_width": ""
4470}
4471},
4472"d861455dc8344b1fbb03c966e8a803ad": {
4473"model_module": "@jupyter-widgets/base",
4474"model_module_version": "1.2.0",
4475"model_name": "LayoutModel",
4476"state": {
4477"_model_module": "@jupyter-widgets/base",
4478"_model_module_version": "1.2.0",
4479"_model_name": "LayoutModel",
4480"_view_count": null,
4481"_view_module": "@jupyter-widgets/base",
4482"_view_module_version": "1.2.0",
4483"_view_name": "LayoutView",
4484"align_content": null,
4485"align_items": null,
4486"align_self": null,
4487"border": null,
4488"bottom": null,
4489"display": null,
4490"flex": null,
4491"flex_flow": null,
4492"grid_area": null,
4493"grid_auto_columns": null,
4494"grid_auto_flow": null,
4495"grid_auto_rows": null,
4496"grid_column": null,
4497"grid_gap": null,
4498"grid_row": null,
4499"grid_template_areas": null,
4500"grid_template_columns": null,
4501"grid_template_rows": null,
4502"height": null,
4503"justify_content": null,
4504"justify_items": null,
4505"left": null,
4506"margin": null,
4507"max_height": null,
4508"max_width": null,
4509"min_height": null,
4510"min_width": null,
4511"object_fit": null,
4512"object_position": null,
4513"order": null,
4514"overflow": null,
4515"overflow_x": null,
4516"overflow_y": null,
4517"padding": null,
4518"right": null,
4519"top": null,
4520"visibility": null,
4521"width": null
4522}
4523},
4524"d8f756ab4c9245709c40a48df7842c07": {
4525"model_module": "@jupyter-widgets/controls",
4526"model_module_version": "1.5.0",
4527"model_name": "ProgressStyleModel",
4528"state": {
4529"_model_module": "@jupyter-widgets/controls",
4530"_model_module_version": "1.5.0",
4531"_model_name": "ProgressStyleModel",
4532"_view_count": null,
4533"_view_module": "@jupyter-widgets/base",
4534"_view_module_version": "1.2.0",
4535"_view_name": "StyleView",
4536"bar_color": null,
4537"description_width": ""
4538}
4539},
4540"dbb57469064a479085473de8e71ce4d0": {
4541"model_module": "@jupyter-widgets/base",
4542"model_module_version": "1.2.0",
4543"model_name": "LayoutModel",
4544"state": {
4545"_model_module": "@jupyter-widgets/base",
4546"_model_module_version": "1.2.0",
4547"_model_name": "LayoutModel",
4548"_view_count": null,
4549"_view_module": "@jupyter-widgets/base",
4550"_view_module_version": "1.2.0",
4551"_view_name": "LayoutView",
4552"align_content": null,
4553"align_items": null,
4554"align_self": null,
4555"border": null,
4556"bottom": null,
4557"display": null,
4558"flex": null,
4559"flex_flow": null,
4560"grid_area": null,
4561"grid_auto_columns": null,
4562"grid_auto_flow": null,
4563"grid_auto_rows": null,
4564"grid_column": null,
4565"grid_gap": null,
4566"grid_row": null,
4567"grid_template_areas": null,
4568"grid_template_columns": null,
4569"grid_template_rows": null,
4570"height": null,
4571"justify_content": null,
4572"justify_items": null,
4573"left": null,
4574"margin": null,
4575"max_height": null,
4576"max_width": null,
4577"min_height": null,
4578"min_width": null,
4579"object_fit": null,
4580"object_position": null,
4581"order": null,
4582"overflow": null,
4583"overflow_x": null,
4584"overflow_y": null,
4585"padding": null,
4586"right": null,
4587"top": null,
4588"visibility": null,
4589"width": null
4590}
4591},
4592"dbd48e8c1a9445e3b757609cf0b60fa3": {
4593"model_module": "@jupyter-widgets/controls",
4594"model_module_version": "1.5.0",
4595"model_name": "DescriptionStyleModel",
4596"state": {
4597"_model_module": "@jupyter-widgets/controls",
4598"_model_module_version": "1.5.0",
4599"_model_name": "DescriptionStyleModel",
4600"_view_count": null,
4601"_view_module": "@jupyter-widgets/base",
4602"_view_module_version": "1.2.0",
4603"_view_name": "StyleView",
4604"description_width": ""
4605}
4606},
4607"ddb287b8aa9c40fbb4bc38b84c2f54d5": {
4608"model_module": "@jupyter-widgets/controls",
4609"model_module_version": "1.5.0",
4610"model_name": "FloatProgressModel",
4611"state": {
4612"_dom_classes": [],
4613"_model_module": "@jupyter-widgets/controls",
4614"_model_module_version": "1.5.0",
4615"_model_name": "FloatProgressModel",
4616"_view_count": null,
4617"_view_module": "@jupyter-widgets/controls",
4618"_view_module_version": "1.5.0",
4619"_view_name": "ProgressView",
4620"bar_style": "success",
4621"description": "",
4622"description_tooltip": null,
4623"layout": "IPY_MODEL_33b5df6b101a4b16bcd0fbb13c8980d9",
4624"max": 1,
4625"min": 0,
4626"orientation": "horizontal",
4627"style": "IPY_MODEL_cf10491e88374c679c94b757ef207144",
4628"value": 1
4629}
4630},
4631"dfe261365e144254ae00ea321579b860": {
4632"model_module": "@jupyter-widgets/controls",
4633"model_module_version": "1.5.0",
4634"model_name": "DescriptionStyleModel",
4635"state": {
4636"_model_module": "@jupyter-widgets/controls",
4637"_model_module_version": "1.5.0",
4638"_model_name": "DescriptionStyleModel",
4639"_view_count": null,
4640"_view_module": "@jupyter-widgets/base",
4641"_view_module_version": "1.2.0",
4642"_view_name": "StyleView",
4643"description_width": ""
4644}
4645},
4646"e19b0f2f1eaf4d58b25e59deee4cc70c": {
4647"model_module": "@jupyter-widgets/controls",
4648"model_module_version": "1.5.0",
4649"model_name": "HBoxModel",
4650"state": {
4651"_dom_classes": [],
4652"_model_module": "@jupyter-widgets/controls",
4653"_model_module_version": "1.5.0",
4654"_model_name": "HBoxModel",
4655"_view_count": null,
4656"_view_module": "@jupyter-widgets/controls",
4657"_view_module_version": "1.5.0",
4658"_view_name": "HBoxView",
4659"box_style": "",
4660"children": [
4661"IPY_MODEL_7ee82a73e82c4ad29a15bf336095fa49",
4662"IPY_MODEL_ddb287b8aa9c40fbb4bc38b84c2f54d5",
4663"IPY_MODEL_9962218c39cf4d20abc2460278fd6808"
4664],
4665"layout": "IPY_MODEL_ed3a7ced6e0247ef93cd20fe3cc62591"
4666}
4667},
4668"e530c06891274d41b5d8a2b20e160ee4": {
4669"model_module": "@jupyter-widgets/controls",
4670"model_module_version": "1.5.0",
4671"model_name": "DescriptionStyleModel",
4672"state": {
4673"_model_module": "@jupyter-widgets/controls",
4674"_model_module_version": "1.5.0",
4675"_model_name": "DescriptionStyleModel",
4676"_view_count": null,
4677"_view_module": "@jupyter-widgets/base",
4678"_view_module_version": "1.2.0",
4679"_view_name": "StyleView",
4680"description_width": ""
4681}
4682},
4683"eaa5a7cf338f4c4faf1f4221684f6fa1": {
4684"model_module": "@jupyter-widgets/base",
4685"model_module_version": "1.2.0",
4686"model_name": "LayoutModel",
4687"state": {
4688"_model_module": "@jupyter-widgets/base",
4689"_model_module_version": "1.2.0",
4690"_model_name": "LayoutModel",
4691"_view_count": null,
4692"_view_module": "@jupyter-widgets/base",
4693"_view_module_version": "1.2.0",
4694"_view_name": "LayoutView",
4695"align_content": null,
4696"align_items": null,
4697"align_self": null,
4698"border": null,
4699"bottom": null,
4700"display": null,
4701"flex": null,
4702"flex_flow": null,
4703"grid_area": null,
4704"grid_auto_columns": null,
4705"grid_auto_flow": null,
4706"grid_auto_rows": null,
4707"grid_column": null,
4708"grid_gap": null,
4709"grid_row": null,
4710"grid_template_areas": null,
4711"grid_template_columns": null,
4712"grid_template_rows": null,
4713"height": null,
4714"justify_content": null,
4715"justify_items": null,
4716"left": null,
4717"margin": null,
4718"max_height": null,
4719"max_width": null,
4720"min_height": null,
4721"min_width": null,
4722"object_fit": null,
4723"object_position": null,
4724"order": null,
4725"overflow": null,
4726"overflow_x": null,
4727"overflow_y": null,
4728"padding": null,
4729"right": null,
4730"top": null,
4731"visibility": null,
4732"width": null
4733}
4734},
4735"ec9801b7916d4911be6cd6c806642d4a": {
4736"model_module": "@jupyter-widgets/controls",
4737"model_module_version": "1.5.0",
4738"model_name": "HBoxModel",
4739"state": {
4740"_dom_classes": [],
4741"_model_module": "@jupyter-widgets/controls",
4742"_model_module_version": "1.5.0",
4743"_model_name": "HBoxModel",
4744"_view_count": null,
4745"_view_module": "@jupyter-widgets/controls",
4746"_view_module_version": "1.5.0",
4747"_view_name": "HBoxView",
4748"box_style": "",
4749"children": [
4750"IPY_MODEL_4402e159d59a4518a894d7ded2025b21",
4751"IPY_MODEL_114859f36d21473e8fb44f4c27a71ca3",
4752"IPY_MODEL_3fa2749682e84da5b073250e391e9970"
4753],
4754"layout": "IPY_MODEL_bbfb9e1799aa4580aef7cbf88d604d84"
4755}
4756},
4757"ed3a7ced6e0247ef93cd20fe3cc62591": {
4758"model_module": "@jupyter-widgets/base",
4759"model_module_version": "1.2.0",
4760"model_name": "LayoutModel",
4761"state": {
4762"_model_module": "@jupyter-widgets/base",
4763"_model_module_version": "1.2.0",
4764"_model_name": "LayoutModel",
4765"_view_count": null,
4766"_view_module": "@jupyter-widgets/base",
4767"_view_module_version": "1.2.0",
4768"_view_name": "LayoutView",
4769"align_content": null,
4770"align_items": null,
4771"align_self": null,
4772"border": null,
4773"bottom": null,
4774"display": null,
4775"flex": null,
4776"flex_flow": null,
4777"grid_area": null,
4778"grid_auto_columns": null,
4779"grid_auto_flow": null,
4780"grid_auto_rows": null,
4781"grid_column": null,
4782"grid_gap": null,
4783"grid_row": null,
4784"grid_template_areas": null,
4785"grid_template_columns": null,
4786"grid_template_rows": null,
4787"height": null,
4788"justify_content": null,
4789"justify_items": null,
4790"left": null,
4791"margin": null,
4792"max_height": null,
4793"max_width": null,
4794"min_height": null,
4795"min_width": null,
4796"object_fit": null,
4797"object_position": null,
4798"order": null,
4799"overflow": null,
4800"overflow_x": null,
4801"overflow_y": null,
4802"padding": null,
4803"right": null,
4804"top": null,
4805"visibility": null,
4806"width": null
4807}
4808},
4809"ef2447b1e70d499ebc080c4e3b46f35c": {
4810"model_module": "@jupyter-widgets/controls",
4811"model_module_version": "1.5.0",
4812"model_name": "DescriptionStyleModel",
4813"state": {
4814"_model_module": "@jupyter-widgets/controls",
4815"_model_module_version": "1.5.0",
4816"_model_name": "DescriptionStyleModel",
4817"_view_count": null,
4818"_view_module": "@jupyter-widgets/base",
4819"_view_module_version": "1.2.0",
4820"_view_name": "StyleView",
4821"description_width": ""
4822}
4823},
4824"ef3fd3ff0c1342958346746f939aa48a": {
4825"model_module": "@jupyter-widgets/base",
4826"model_module_version": "1.2.0",
4827"model_name": "LayoutModel",
4828"state": {
4829"_model_module": "@jupyter-widgets/base",
4830"_model_module_version": "1.2.0",
4831"_model_name": "LayoutModel",
4832"_view_count": null,
4833"_view_module": "@jupyter-widgets/base",
4834"_view_module_version": "1.2.0",
4835"_view_name": "LayoutView",
4836"align_content": null,
4837"align_items": null,
4838"align_self": null,
4839"border": null,
4840"bottom": null,
4841"display": null,
4842"flex": null,
4843"flex_flow": null,
4844"grid_area": null,
4845"grid_auto_columns": null,
4846"grid_auto_flow": null,
4847"grid_auto_rows": null,
4848"grid_column": null,
4849"grid_gap": null,
4850"grid_row": null,
4851"grid_template_areas": null,
4852"grid_template_columns": null,
4853"grid_template_rows": null,
4854"height": null,
4855"justify_content": null,
4856"justify_items": null,
4857"left": null,
4858"margin": null,
4859"max_height": null,
4860"max_width": null,
4861"min_height": null,
4862"min_width": null,
4863"object_fit": null,
4864"object_position": null,
4865"order": null,
4866"overflow": null,
4867"overflow_x": null,
4868"overflow_y": null,
4869"padding": null,
4870"right": null,
4871"top": null,
4872"visibility": null,
4873"width": null
4874}
4875},
4876"f12d6748a4bc478c92d8e8ed8730cce2": {
4877"model_module": "@jupyter-widgets/controls",
4878"model_module_version": "1.5.0",
4879"model_name": "FloatProgressModel",
4880"state": {
4881"_dom_classes": [],
4882"_model_module": "@jupyter-widgets/controls",
4883"_model_module_version": "1.5.0",
4884"_model_name": "FloatProgressModel",
4885"_view_count": null,
4886"_view_module": "@jupyter-widgets/controls",
4887"_view_module_version": "1.5.0",
4888"_view_name": "ProgressView",
4889"bar_style": "success",
4890"description": "",
4891"description_tooltip": null,
4892"layout": "IPY_MODEL_7165f68ae5fd4b57a3f30942519f2d29",
4893"max": 1,
4894"min": 0,
4895"orientation": "horizontal",
4896"style": "IPY_MODEL_4c41e83814e44a578a6e62585cafd34d",
4897"value": 1
4898}
4899},
4900"f39c9f27960e4ff99bd7aef02f14ffd2": {
4901"model_module": "@jupyter-widgets/controls",
4902"model_module_version": "1.5.0",
4903"model_name": "DescriptionStyleModel",
4904"state": {
4905"_model_module": "@jupyter-widgets/controls",
4906"_model_module_version": "1.5.0",
4907"_model_name": "DescriptionStyleModel",
4908"_view_count": null,
4909"_view_module": "@jupyter-widgets/base",
4910"_view_module_version": "1.2.0",
4911"_view_name": "StyleView",
4912"description_width": ""
4913}
4914},
4915"f54f6e8998f54d5c8d367d244158c261": {
4916"model_module": "@jupyter-widgets/controls",
4917"model_module_version": "1.5.0",
4918"model_name": "DescriptionStyleModel",
4919"state": {
4920"_model_module": "@jupyter-widgets/controls",
4921"_model_module_version": "1.5.0",
4922"_model_name": "DescriptionStyleModel",
4923"_view_count": null,
4924"_view_module": "@jupyter-widgets/base",
4925"_view_module_version": "1.2.0",
4926"_view_name": "StyleView",
4927"description_width": ""
4928}
4929},
4930"f6bac06384a84aea8a470bf612e90d2a": {
4931"model_module": "@jupyter-widgets/controls",
4932"model_module_version": "1.5.0",
4933"model_name": "DescriptionStyleModel",
4934"state": {
4935"_model_module": "@jupyter-widgets/controls",
4936"_model_module_version": "1.5.0",
4937"_model_name": "DescriptionStyleModel",
4938"_view_count": null,
4939"_view_module": "@jupyter-widgets/base",
4940"_view_module_version": "1.2.0",
4941"_view_name": "StyleView",
4942"description_width": ""
4943}
4944},
4945"f73977385dce49bead6c2012f2e42f71": {
4946"model_module": "@jupyter-widgets/controls",
4947"model_module_version": "1.5.0",
4948"model_name": "HTMLModel",
4949"state": {
4950"_dom_classes": [],
4951"_model_module": "@jupyter-widgets/controls",
4952"_model_module_version": "1.5.0",
4953"_model_name": "HTMLModel",
4954"_view_count": null,
4955"_view_module": "@jupyter-widgets/controls",
4956"_view_module_version": "1.5.0",
4957"_view_name": "HTMLView",
4958"description": "",
4959"description_tooltip": null,
4960"layout": "IPY_MODEL_bd2fb1c05642482bbed1e4f4e2824f80",
4961"placeholder": "",
4962"style": "IPY_MODEL_5b64bdd8ce404f8290511d9bf9c7f29f",
4963"value": " 1/1 [00:00<00:00, 17.25it/s]"
4964}
4965},
4966"f76772d14e4a4f0487044dcbd253f82e": {
4967"model_module": "@jupyter-widgets/controls",
4968"model_module_version": "1.5.0",
4969"model_name": "HBoxModel",
4970"state": {
4971"_dom_classes": [],
4972"_model_module": "@jupyter-widgets/controls",
4973"_model_module_version": "1.5.0",
4974"_model_name": "HBoxModel",
4975"_view_count": null,
4976"_view_module": "@jupyter-widgets/controls",
4977"_view_module_version": "1.5.0",
4978"_view_name": "HBoxView",
4979"box_style": "",
4980"children": [
4981"IPY_MODEL_c321ff97706d465193a2b3dfcb107c1c",
4982"IPY_MODEL_842019f6aed44d41976b11a0352d4596",
4983"IPY_MODEL_729a5070b09f4b2aa511d58e2b5833d5"
4984],
4985"layout": "IPY_MODEL_8fb5aba463ac40a18063b4fc7cd3d879"
4986}
4987},
4988"fd2f080261c74992bd7fb9a0b6f6010b": {
4989"model_module": "@jupyter-widgets/controls",
4990"model_module_version": "1.5.0",
4991"model_name": "HBoxModel",
4992"state": {
4993"_dom_classes": [],
4994"_model_module": "@jupyter-widgets/controls",
4995"_model_module_version": "1.5.0",
4996"_model_name": "HBoxModel",
4997"_view_count": null,
4998"_view_module": "@jupyter-widgets/controls",
4999"_view_module_version": "1.5.0",
5000"_view_name": "HBoxView",
5001"box_style": "",
5002"children": [
5003"IPY_MODEL_7fd49ced837540d2b3e560a6dd507ac3",
5004"IPY_MODEL_7f69e47ac44c401f9b26f8c88de4c481",
5005"IPY_MODEL_0466c5b6e97b467b844ff2b72ab9de8e"
5006],
5007"layout": "IPY_MODEL_6baf2644608242a6a431cd3939201d51"
5008}
5009}
5010}
5011}
5012},
5013"nbformat": 4,
5014"nbformat_minor": 0
5015}
5016